4) 以下是一个List类模板的定义: 模板<类T>类列表{ 公共: List();//构造函数 void Add(T&);//在Link表头添加新结点 void Remove(T&);//在Link中删除含有特定值的元素 T*Find(T&);//查找含有特定值的结点 void PrintList();//打印输出整个链表 ~列表(); 受保护的: 结构节点{ 节点* pNext; T * pT; }; 节点* pFirst; //链首结点指针 }; 完成对上述List类模板含有的各成员函数的定义。然后定义一个简单的Student类,并 利用编写的List类模板对一个班级的学生进行动态管理。(根据自己的能力选做)。
时间: 2024-02-12 11:07:58 浏览: 115
以下是List类模板的各成员函数的定义:
```c++
template<class T>
class List {
public:
List() : pFirst(nullptr) {} //构造函数
void Add(T& t) { //在Link表头添加新结点
Node* pNode = new Node;
pNode->pT = &t;
pNode->pNext = pFirst;
pFirst = pNode;
}
void Remove(T& t) { //在Link中删除含有特定值的元素
Node* pNode = pFirst;
Node* pPrev = nullptr;
while (pNode) {
if (*(pNode->pT) == t) {
if (pPrev) {
pPrev->pNext = pNode->pNext;
} else {
pFirst = pNode->pNext;
}
delete pNode;
return;
}
pPrev = pNode;
pNode = pNode->pNext;
}
}
T* Find(T& t) { //查找含有特定值的结点
Node* pNode = pFirst;
while (pNode) {
if (*(pNode->pT) == t) {
return pNode->pT;
}
pNode = pNode->pNext;
}
return nullptr;
}
void PrintList() { //打印输出整个链表
Node* pNode = pFirst;
while (pNode) {
std::cout << *(pNode->pT) << std::endl;
pNode = pNode->pNext;
}
}
~List() { //析构函数
Node* pNode = pFirst;
while (pNode) {
Node* pNext = pNode->pNext;
delete pNode;
pNode = pNext;
}
}
protected:
struct Node { //节点结构体
Node* pNext;
T* pT;
};
Node* pFirst; //链首结点指针
};
```
接下来,我们可以定义一个简单的Student类,并利用编写的List类模板对一个班级的学生进行动态管理,如下:
```c++
#include <iostream>
#include <string>
using namespace std;
class Student {
public:
Student(string name = "", int age = 0, int score = 0)
: name(name), age(age), score(score) {}
bool operator==(const Student& s) const {
return name == s.name && age == s.age && score == s.score;
}
friend ostream& operator<<(ostream& os, const Student& s) {
os << "name: " << s.name << ", age: " << s.age << ", score: " << s.score;
return os;
}
private:
string name;
int age;
int score;
};
int main() {
List<Student> stuList;
stuList.Add(Student("Alice", 18, 90));
stuList.Add(Student("Bob", 19, 85));
stuList.Add(Student("Charlie", 20, 95));
cout << "students in class:" << endl;
stuList.PrintList();
Student s("Bob", 19, 85);
cout << "find student: " << s << endl;
Student* p = stuList.Find(s);
if (p) {
cout << "found: " << *p << endl;
} else {
cout << "not found" << endl;
}
stuList.Remove(s);
cout << "after remove " << s << ":" << endl;
stuList.PrintList();
return 0;
}
```
阅读全文
相关推荐
















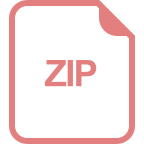

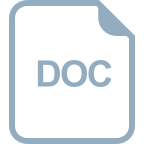