以下是一个List类模板的定义: 模板<类T>类列表{ 公共: List();//构造函数 void Add(T&);//在Link表头添加新结点 void Remove(T&);//在Link中删除含有特定值的元素 T*Find(T&);//查找含有特定值的结点 void PrintList();//打印输出整个链表 ~列表(); 受保护的: 结构节点{ 节点* pNext; T * pT; }; 节点* pFirst; //链首结点指针 }; 完成对上述List类模板含有的各成员函数的定义。然后定义一个简单的Student类,并 利用编写的List类模板对一个班级的学生进行动态管理。(根据自己的能力选做)。
时间: 2024-02-12 15:09:14 浏览: 26
下面是List类模板各成员函数的定义:
```cpp
template<class T>
class List{
public:
List():pFirst(nullptr){} //构造函数
void Add(T& val){
Node* pNode = new Node;
pNode->pT = &val;
pNode->pNext = pFirst;
pFirst = pNode;
} //在Link表头添加新结点
void Remove(T& val){
Node* pNode = pFirst;
Node* pPrev = nullptr;
while(pNode){
if(*(pNode->pT) == val){
if(pPrev){
pPrev->pNext = pNode->pNext;
}else{
pFirst = pNode->pNext;
}
delete pNode;
return;
}
pPrev = pNode;
pNode = pNode->pNext;
}
} //在Link中删除含有特定值的元素
T* Find(T& val){
Node* pNode = pFirst;
while(pNode){
if(*(pNode->pT) == val){
return pNode->pT;
}
pNode = pNode->pNext;
}
return nullptr;
} //查找含有特定值的结点
void PrintList(){
Node* pNode = pFirst;
while(pNode){
std::cout << *(pNode->pT) << std::endl;
pNode = pNode->pNext;
}
} //打印输出整个链表
~List(){
Node* pNode = pFirst;
while(pNode){
Node* pNext = pNode->pNext;
delete pNode;
pNode = pNext;
}
}
protected:
struct Node{
Node* pNext;
T* pT;
};
Node* pFirst; //链首结点指针
};
```
下面是简单的Student类定义:
```cpp
class Student{
public:
Student(const std::string& name, int id):name_(name), id_(id){}
bool operator==(const Student& other) const{
return id_ == other.id_;
}
friend std::ostream& operator<<(std::ostream& os, const Student& student);
private:
std::string name_;
int id_;
};
std::ostream& operator<<(std::ostream& os, const Student& student){
os << "Name: " << student.name_ << ", ID: " << student.id_;
return os;
}
```
下面是利用List类模板对一个班级的学生进行动态管理的示例代码:
```cpp
int main(){
List<Student> classList;
Student s1("Alice", 1001);
Student s2("Bob", 1002);
Student s3("Charlie", 1003);
//添加学生
classList.Add(s1);
classList.Add(s2);
classList.Add(s3);
//打印学生
classList.PrintList();
std::cout << std::endl;
//查找学生
Student s4("Bob", 1002);
Student* pStudent = classList.Find(s4);
if(pStudent){
std::cout << "Found student: " << *pStudent << std::endl;
}else{
std::cout << "Student not found." << std::endl;
}
std::cout << std::endl;
//删除学生
classList.Remove(s2);
classList.PrintList();
return 0;
}
```
相关推荐
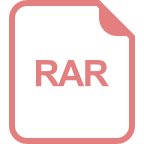
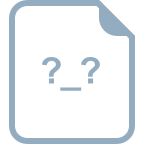
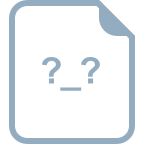














