写一个解压gz格式文件的C程序
时间: 2023-09-07 13:13:32 浏览: 133
以下是一个使用C语言进行解压gz格式文件的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <zlib.h>
#define CHUNK_SIZE 16384 // 解压缓冲区大小
int decompress_gz_file(const char *gz_file_path, const char *output_file_path) {
int ret;
unsigned have;
z_stream strm;
unsigned char in[CHUNK_SIZE];
unsigned char out[CHUNK_SIZE];
FILE *gz_file = NULL, *output_file = NULL;
// 打开gz文件
gz_file = fopen(gz_file_path, "rb");
if (gz_file == NULL) {
fprintf(stderr, "Failed to open gz file: %s\n", gz_file_path);
return -1;
}
// 打开输出文件
output_file = fopen(output_file_path, "wb");
if (output_file == NULL) {
fprintf(stderr, "Failed to open output file: %s\n", output_file_path);
fclose(gz_file);
return -1;
}
// 初始化zlib解压器
memset(&strm, 0, sizeof(strm));
ret = inflateInit2(&strm, MAX_WBITS + 16);
if (ret != Z_OK) {
fprintf(stderr, "Failed to initialize zlib: %s\n", strm.msg);
fclose(output_file);
fclose(gz_file);
return -1;
}
// 开始解压
do {
strm.avail_in = fread(in, 1, CHUNK_SIZE, gz_file);
if (ferror(gz_file)) {
fprintf(stderr, "Failed to read gz file: %s\n", gz_file_path);
inflateEnd(&strm);
fclose(output_file);
fclose(gz_file);
return -1;
}
if (strm.avail_in == 0) {
break;
}
strm.next_in = in;
do {
strm.avail_out = CHUNK_SIZE;
strm.next_out = out;
ret = inflate(&strm, Z_NO_FLUSH);
switch (ret) {
case Z_NEED_DICT:
case Z_DATA_ERROR:
case Z_MEM_ERROR:
inflateEnd(&strm);
fclose(output_file);
fclose(gz_file);
return -1;
}
have = CHUNK_SIZE - strm.avail_out;
if (fwrite(out, 1, have, output_file) != have || ferror(output_file)) {
fprintf(stderr, "Failed to write output file: %s\n", output_file_path);
inflateEnd(&strm);
fclose(output_file);
fclose(gz_file);
return -1;
}
} while (strm.avail_out == 0);
} while (ret != Z_STREAM_END);
// 结束解压
inflateEnd(&strm);
// 关闭文件
fclose(output_file);
fclose(gz_file);
return 0;
}
// 示例用法
int main() {
decompress_gz_file("example.gz", "example.txt");
return 0;
}
```
以上程序中,`decompress_gz_file` 函数接受两个参数,第一个参数 `gz_file_path` 是需要解压的gz文件的路径,第二个参数 `output_file_path` 是解压后输出文件的路径。
程序使用 zlib 库中的 `inflate` 函数解压gz文件。程序首先打开gz文件和输出文件,然后初始化zlib解压器,接着不断读取gz文件中的数据并解压,最后将解压后的数据写入到输出文件中,直到解压完成。
注意:以上示例程序仅适用于解压单个文件,如果需要解压包含多个文件的gz压缩文件,需要使用其他工具或库进行解压。
相关推荐
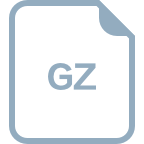
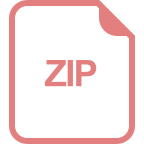
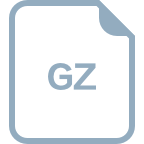














