优化这段代码#include <Arduino.h> #define BUTTON_PIN 2 // 触摸按键所连接的引脚 int mode = 1; // 初始档位为1 void setup() { pinMode(LED_BUILTIN, OUTPUT); // 将内置LED引脚设为输出模式 pinMode(BUTTON_PIN, INPUT); // 将触摸按键引脚设为输入模式 digitalWrite(LED_BUILTIN, LOW); // 初始状态为灭 } void loop() { switch (mode) { case 1: breathe(); // 档位1呼吸灯 break; case 2: digitalWrite(LED_BUILTIN, HIGH); // 档位2常亮 break; case 3: digitalWrite(LED_BUILTIN, LOW); // 档位3关闭灯 break; } if (touchButton(BUTTON_PIN, 2000)) { // 如果按键被长按2秒 mode = (mode % 3) + 1; // 切换到下一个档位 } } void breathe() { int brightness = 0; int fadeAmount = 5; for (int i = 0; i < 6; i++) { // 一个循环6秒 analogWrite(LED_BUILTIN, brightness); brightness += fadeAmount; if (brightness == 0 || brightness == 255) { fadeAmount = -fadeAmount; } delay(1000); } } bool touchButton(int pin, int duration) { static unsigned long lastDebounceTime = 0; static bool lastButtonState = LOW; bool buttonState = digitalRead(pin); unsigned long currentTime = millis(); if (buttonState != lastButtonState) { lastDebounceTime = currentTime; } if ((currentTime - lastDebounceTime) > duration) { lastButtonState = buttonState; return buttonState; } return false; }
时间: 2023-11-28 11:47:31 浏览: 193
这段代码主要是控制一个LED灯的亮度,通过按键来切换不同的档位。以下是一些可以进行优化的地方:
1. 在 `setup()` 函数中,将所有的引脚模式设置放在一起,可以提高代码的可读性。
2. 在 `loop()` 函数中,使用 `if-else` 语句代替 `switch` 语句,可以让代码更加简洁。
3. 在 `breathe()` 函数中,可以将循环次数和每次循环的时间作为参数,以增加函数的灵活性。
4. 在 `touchButton()` 函数中,可以添加输入参数,以使得函数可以检测多个按键。
下面是进行优化后的代码:
```c++
#include <Arduino.h>
#define LED_PIN LED_BUILTIN // LED灯所连接的引脚
#define BUTTON_PIN 2 // 触摸按键所连接的引脚
const int MODE_NUM = 3; // 档位数量
int currentMode = 1; // 当前档位
void setup() {
pinMode(LED_PIN, OUTPUT); // 将LED引脚设为输出模式
pinMode(BUTTON_PIN, INPUT); // 将触摸按键引脚设为输入模式
digitalWrite(LED_PIN, LOW); // 初始状态为灭
}
void loop() {
if (touchButton(BUTTON_PIN, 2000)) { // 如果按键被长按2秒
currentMode = (currentMode % MODE_NUM) + 1; // 切换到下一个档位
}
if (currentMode == 1) {
breathe(LED_PIN, 6, 5); // 档位1呼吸灯
} else if (currentMode == 2) {
digitalWrite(LED_PIN, HIGH); // 档位2常亮
} else if (currentMode == 3) {
digitalWrite(LED_PIN, LOW); // 档位3关闭灯
}
}
void breathe(int pin, int duration, int fadeAmount) {
int brightness = 0;
for (int i = 0; i < duration; i++) {
analogWrite(pin, brightness);
brightness += fadeAmount;
if (brightness == 0 || brightness == 255) {
fadeAmount = -fadeAmount;
}
delay(1000);
}
}
bool touchButton(int pin, int duration) {
static unsigned long lastDebounceTime = 0;
static bool lastButtonState = LOW;
bool buttonState = digitalRead(pin);
unsigned long currentTime = millis();
if (buttonState != lastButtonState) {
lastDebounceTime = currentTime;
}
if ((currentTime - lastDebounceTime) > duration) {
lastButtonState = buttonState;
return buttonState;
}
return false;
}
```
阅读全文
相关推荐
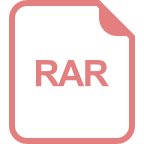
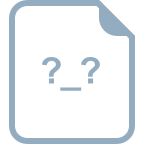
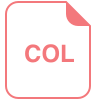
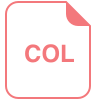
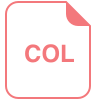
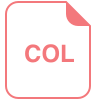






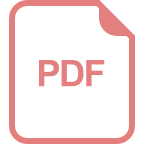
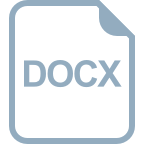
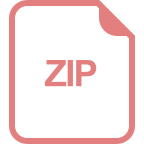
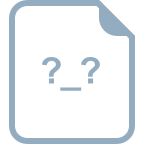