C#什么将字符串“(dgg)(dagg)(d1gg)(d2gg)”括号里面的字符一个个取出来
时间: 2023-11-24 15:05:24 浏览: 34
在C#中,你可以使用正则表达式或者字符串操作来将括号里面的字符一个个取出来。以下是两种方法的示例代码:
方法一:使用正则表达式和`Regex`类
```csharp
using System;
using System.Text.RegularExpressions;
public class Program
{
public static void Main(string[] args)
{
string str = "(dgg)(dagg)(d1gg)(d2gg)";
Regex regex = new Regex(@"\((.*?)\)");
MatchCollection matches = regex.Matches(str);
foreach (Match match in matches)
{
string content = match.Groups[1].Value;
Console.WriteLine(content);
}
}
}
```
运行以上代码,会输出以下结果:
```
dgg
dagg
d1gg
d2gg
```
使用正则表达式`\((.*?)\)`可以匹配括号内的内容,通过`match.Groups[1].Value`可以获取括号内的字符内容。遍历`MatchCollection`对象可以逐个取出括号内的字符。
方法二:使用字符串操作
```csharp
using System;
public class Program
{
public static void Main(string[] args)
{
string str = "(dgg)(dagg)(d1gg)(d2gg)";
int startIndex = str.IndexOf('(');
while (startIndex >= 0)
{
int endIndex = str.IndexOf(')', startIndex);
if (endIndex < 0)
{
break;
}
string content = str.Substring(startIndex + 1, endIndex - startIndex - 1);
Console.WriteLine(content);
startIndex = str.IndexOf('(', endIndex);
}
}
}
```
运行以上代码,会输出以下结果:
```
dgg
dagg
d1gg
d2gg
```
使用`IndexOf()`方法找到括号的起始位置和结束位置,然后使用`Substring()`方法提取括号内的字符内容。循环遍历可以逐个取出括号内的字符。
相关推荐
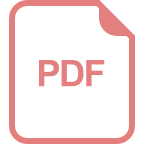
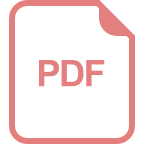
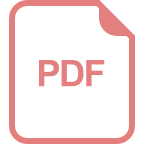




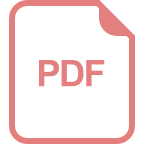
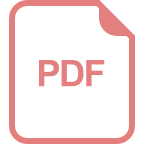
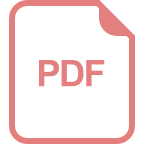
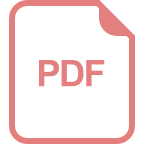
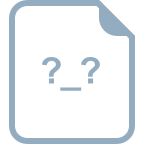