这样判断共面是错误的,请修正使用c++实现
时间: 2024-03-27 07:39:25 浏览: 16
判断四个点是否共面可以通过计算它们的行列式值来实现。具体来说,如果四个点都在同一个平面上,则它们的行列式值为0。
以下是使用 C++ 实现的代码示例:
```c++
#include <iostream>
using namespace std;
struct Point {
double x, y, z;
};
// 计算行列式值
double det(double a[4][4]) {
double sum = 0;
for (int i = 0; i < 4; i++) {
sum += a[0][i] * (a[1][(i+1)%4] * (a[2][(i+2)%4] * a[3][(i+3)%4] - a[2][(i+3)%4] * a[3][(i+2)%4]) - a[1][(i+2)%4] * (a[2][(i+1)%4] * a[3][(i+3)%4] - a[2][(i+3)%4] * a[3][(i+1)%4]) + a[1][(i+3)%4] * (a[2][(i+1)%4] * a[3][(i+2)%4] - a[2][(i+2)%4] * a[3][(i+1)%4]));
}
return sum;
}
// 判断四个点是否共面
bool isCoplanar(Point a, Point b, Point c, Point d) {
double m[4][4] = {
{a.x, a.y, a.z, 1},
{b.x, b.y, b.z, 1},
{c.x, c.y, c.z, 1},
{d.x, d.y, d.z, 1}
};
return det(m) == 0;
}
int main() {
Point a = {0, 0, 0};
Point b = {1, 1, 1};
Point c = {2, 2, 2};
Point d = {3, 3, 3};
if (isCoplanar(a, b, c, d)) {
cout << "四个点共面" << endl;
} else {
cout << "四个点不共面" << endl;
}
return 0;
}
```
在这个例子中,我们定义了一个 `Point` 结构体来表示三维空间中的一个点。`det` 函数用于计算一个 4x4 的矩阵的行列式值。在 `isCoplanar` 函数中,我们将四个点的坐标作为矩阵的元素,然后调用 `det` 函数来计算行列式值是否为0,从而判断四个点是否共面。最后,我们在主函数中调用 `isCoplanar` 函数来检查四个点是否共面。
相关推荐
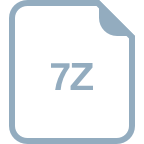
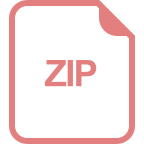















