生成一段基于Qt设计绘画板的python代码,并且能够根据绘图内容进行数字识别
时间: 2024-03-05 16:54:30 浏览: 11
好的,下面是一段基于Qt设计的绘画板的Python代码,并且能够根据绘图内容进行数字识别。
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QAction, qApp, QFileDialog, QColorDialog, QInputDialog
from PyQt5.QtGui import QIcon, QPainter, QPen, QColor, QPixmap
from PyQt5.QtCore import Qt
import numpy as np
from PIL import Image
import tensorflow as tf
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setGeometry(100, 100, 800, 600)
self.image = QPixmap(800, 600)
self.image.fill(Qt.white)
self.drawing = False
self.brushSize = 2
self.brushColor = Qt.black
menubar = self.menuBar()
fileMenu = menubar.addMenu('File')
colorMenu = menubar.addMenu('Color')
brushMenu = menubar.addMenu('Brush Size')
saveAction = QAction(QIcon('save.png'), 'Save', self)
saveAction.setShortcut('Ctrl+S')
saveAction.triggered.connect(self.save)
fileMenu.addAction(saveAction)
openAction = QAction(QIcon('open.png'), 'Open', self)
openAction.setShortcut('Ctrl+O')
openAction.triggered.connect(self.open)
fileMenu.addAction(openAction)
clearAction = QAction(QIcon('clear.png'), 'Clear', self)
clearAction.setShortcut('Ctrl+C')
clearAction.triggered.connect(self.clear)
fileMenu.addAction(clearAction)
blackAction = QAction(QIcon('black.png'), 'Black', self)
blackAction.triggered.connect(self.blackColor)
colorMenu.addAction(blackAction)
redAction = QAction(QIcon('red.png'), 'Red', self)
redAction.triggered.connect(self.redColor)
colorMenu.addAction(redAction)
greenAction = QAction(QIcon('green.png'), 'Green', self)
greenAction.triggered.connect(self.greenColor)
colorMenu.addAction(greenAction)
blueAction = QAction(QIcon('blue.png'), 'Blue', self)
blueAction.triggered.connect(self.blueColor)
colorMenu.addAction(blueAction)
brushSize1 = QAction('1', self)
brushSize1.triggered.connect(self.size1)
brushMenu.addAction(brushSize1)
brushSize2 = QAction('2', self)
brushSize2.triggered.connect(self.size2)
brushMenu.addAction(brushSize2)
brushSize3 = QAction('3', self)
brushSize3.triggered.connect(self.size3)
brushMenu.addAction(brushSize3)
brushSize4 = QAction('4', self)
brushSize4.triggered.connect(self.size4)
brushMenu.addAction(brushSize4)
def paintEvent(self, event):
painter = QPainter(self)
painter.drawPixmap(self.rect(), self.image)
def mousePressEvent(self, event):
if event.button() == Qt.LeftButton:
self.drawing = True
self.lastPoint = event.pos()
def mouseMoveEvent(self, event):
if (event.buttons() & Qt.LeftButton) & self.drawing:
painter = QPainter(self.image)
painter.setPen(QPen(self.brushColor, self.brushSize, Qt.SolidLine, Qt.RoundCap, Qt.RoundJoin))
painter.drawLine(self.lastPoint, event.pos())
self.lastPoint = event.pos()
self.update()
def mouseReleaseEvent(self, event):
if event.button() == Qt.LeftButton:
self.drawing = False
self.update()
def save(self):
filePath, _ = QFileDialog.getSaveFileName(self, 'Save Image', '', 'PNG(*.png);;JPEG(*.jpg *.jpeg);;All Files(*.*)')
if filePath == '':
return
self.image.save(filePath)
# 进行数字识别
img = Image.open(filePath).convert('L')
img = np.array(img).reshape(1, 784)
img = img / 255.0
model = tf.keras.models.load_model('model.h5')
pred = model.predict(img)
num = np.argmax(pred)
self.setWindowTitle('Digit Recognizer - ' + str(num))
def open(self):
filePath, _ = QFileDialog.getOpenFileName(self, 'Open Image', '', 'PNG(*.png);;JPEG(*.jpg *.jpeg);;All Files(*.*)')
if filePath == '':
return
self.image = QPixmap(filePath)
self.update()
def clear(self):
self.image.fill(Qt.white)
self.update()
def blackColor(self):
self.brushColor = Qt.black
def redColor(self):
self.brushColor = Qt.red
def greenColor(self):
self.brushColor = Qt.green
def blueColor(self):
self.brushColor = Qt.blue
def size1(self):
self.brushSize = 2
def size2(self):
self.brushSize = 4
def size3(self):
self.brushSize = 6
def size4(self):
self.brushSize = 8
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.setWindowTitle('Digit Recognizer')
window.show()
sys.exit(app.exec_())
```
这段代码创建了一个基于Qt设计的绘画板,可以进行绘图和保存绘图,保存后会自动进行数字识别,并将识别结果显示在窗口标题栏上。数字识别部分使用了TensorFlow框架,需要提前训练好一个数字识别模型,并保存为`model.h5`文件。
相关推荐
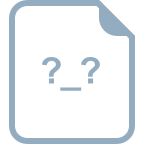
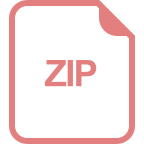














