用pytorch写一个Deeplab v3+网络,把其中的DCNN模块替换为金字塔卷积模块
时间: 2023-02-22 09:40:05 浏览: 106
好的,我可以提供一个基于 PyTorch 的 DeepLab v3 网络的代码框架,并将其中的 DCNN 模块替换为金字塔卷积模块。
首先,需要定义金字塔卷积模块。下面是一个示例代码:
```
import torch
import torch.nn as nn
class PyramidConv(nn.Module):
def __init__(self, in_channels, out_channels, stride=1):
super(PyramidConv, self).__init__()
self.conv1 = nn.Conv2d(in_channels, out_channels, kernel_size=3, stride=stride, padding=1)
self.bn1 = nn.BatchNorm2d(out_channels)
self.conv2 = nn.Conv2d(out_channels, out_channels, kernel_size=3, stride=1, padding=1)
self.bn2 = nn.BatchNorm2d(out_channels)
def forward(self, x):
x = self.conv1(x)
x = self.bn1(x)
x = torch.relu(x)
x = self.conv2(x)
x = self.bn2(x)
x = torch.relu(x)
return x
```
然后,在 DeepLab v3 网络中使用金字塔卷积模块:
```
import torch
import torch.nn as nn
class DeepLabV3(nn.Module):
def __init__(self, num_classes=21):
super(DeepLabV3, self).__init__()
self.backbone = nn.Sequential(
nn.Conv2d(3, 64, kernel_size=3, stride=2, padding=1),
nn.BatchNorm2d(64),
nn.ReLU(),
nn.Conv2d(64, 128, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(128),
nn.ReLU(),
PyramidConv(128, 256, stride=2),
PyramidConv(256, 512),
PyramidConv(512, 1024),
)
self.fc = nn.Sequential(
nn.Conv2d(1024, num_classes, kernel_size=1),
nn.Upsample(scale_factor=8, mode='bilinear', align_corners=True)
)
def forward(self
阅读全文
相关推荐
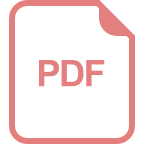



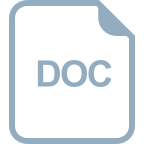

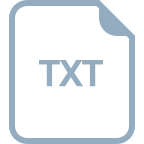
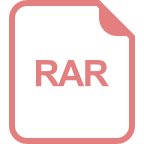
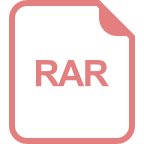
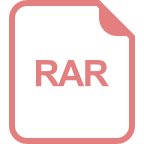
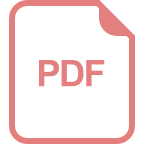
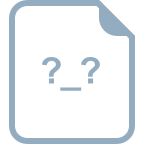
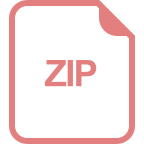
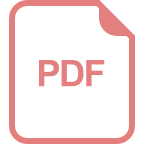
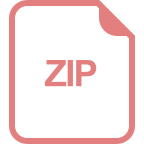
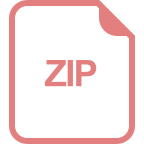
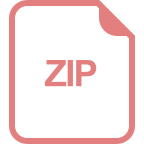