c语言实现将一个数组中元素用来创建二叉排序树
时间: 2024-02-12 22:04:57 浏览: 32
下面是将一个数组中的元素用来创建二叉排序树的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node* left;
struct node* right;
} Node;
Node* create_node(int data) {
Node* new_node = (Node*) malloc(sizeof(Node));
new_node->data = data;
new_node->left = NULL;
new_node->right = NULL;
return new_node;
}
Node* insert(Node* root, int data) {
if (root == NULL) {
return create_node(data);
}
if (data < root->data) {
root->left = insert(root->left, data);
} else {
root->right = insert(root->right, data);
}
return root;
}
void inorder(Node* root) {
if (root == NULL) {
return;
}
inorder(root->left);
printf("%d ", root->data);
inorder(root->right);
}
int main() {
int arr[] = { 50, 30, 70, 20, 40, 60, 80 };
int n = sizeof(arr) / sizeof(arr[0]);
Node* root = NULL;
for (int i = 0; i < n; i++) {
root = insert(root, arr[i]);
}
printf("Inorder traversal of the BST: ");
inorder(root);
printf("\n");
return 0;
}
```
这段代码中,我们定义了一个二叉树节点结构体,包含一个整数类型的数据域,以及指向左右子节点的指针。我们通过create_node函数创建一个新节点,通过insert函数将数组中的元素插入到二叉排序树中。在main函数中,我们先定义了一个整型数组arr,并计算出数组的长度n。然后,我们初始化根节点为NULL,并使用for循环将数组中的元素一个个插入到二叉排序树中。最后,我们通过inorder函数实现了中序遍历,以便对整个二叉排序树进行遍历和输出。
相关推荐
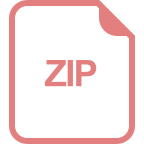
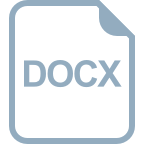
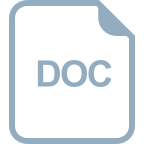















