c语言输入10个不同整数,依次插入到为空的二叉排序树中,并对其进行中序遍历的代码
时间: 2023-12-06 09:45:14 浏览: 38
下面是C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
struct TreeNode* createNode(int val) {
struct TreeNode* node = (struct TreeNode*)malloc(sizeof(struct TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
void insertNode(struct TreeNode* root, int val) {
if(val < root->val) {
if(root->left) {
insertNode(root->left, val);
} else {
root->left = createNode(val);
}
} else {
if(root->right) {
insertNode(root->right, val);
} else {
root->right = createNode(val);
}
}
}
void inorderTraversal(struct TreeNode* root) {
if(!root) return;
inorderTraversal(root->left);
printf("%d ", root->val);
inorderTraversal(root->right);
}
int main() {
int nums[10] = {2, 4, 1, 8, 3, 6, 5, 9, 7, 0};
struct TreeNode* root = createNode(nums[0]);
for(int i = 1; i < 10; i++) {
insertNode(root, nums[i]);
}
inorderTraversal(root);
return 0;
}
```
该程序首先创建一个二叉排序树的根节点,然后遍历输入数组,将每个元素插入到树中。最后对树进行中序遍历并输出结果。
相关推荐
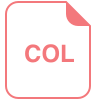
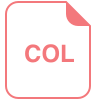
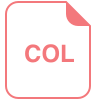
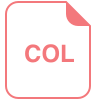
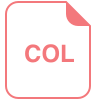









