输入10个不同整数,依次插入到一颗初始为空的二叉排序树中,并对其进行中序遍历,以验证树的正确性的c语言代码
时间: 2024-02-09 11:13:04 浏览: 23
好的,以下是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
// 向二叉树中插入节点
struct TreeNode* insert(struct TreeNode* root, int val) {
if (!root) {
root = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->val = val;
root->left = NULL;
root->right = NULL;
} else if (val < root->val) {
root->left = insert(root->left, val);
} else if (val > root->val) {
root->right = insert(root->right, val);
}
return root;
}
// 中序遍历二叉树
void inorder(struct TreeNode* root) {
if (root) {
inorder(root->left);
printf("%d ", root->val);
inorder(root->right);
}
}
int main() {
// 输入10个不同整数
int nums[10];
for (int i = 0; i < 10; i++) {
scanf("%d", &nums[i]);
}
// 依次插入到一颗初始为空的二叉排序树中
struct TreeNode* root = NULL;
for (int i = 0; i < 10; i++) {
root = insert(root, nums[i]);
}
// 对其进行中序遍历,以验证树的正确性
inorder(root);
return 0;
}
```
你可以将这段代码复制到你的 C 语言环境中编译运行,并输入 10 个不同的整数,程序会输出它们的中序遍历结果,以验证树的正确性。
相关推荐
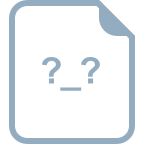
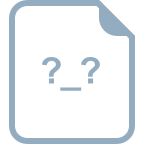










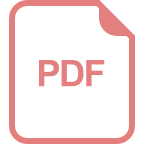