输入10个不同整数,依次插入到一颗初始为空的二叉排序树中,并对其进行中序遍历,以验证树的正确性。c语言
时间: 2024-02-13 18:04:12 浏览: 32
好的,下面是C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树结点结构体
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 二叉搜索树的插入操作
TreeNode* insert(TreeNode* root, int val) {
if (root == NULL) {
root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = val;
root->left = NULL;
root->right = NULL;
} else if (val < root->val) {
root->left = insert(root->left, val);
} else {
root->right = insert(root->right, val);
}
return root;
}
// 中序遍历二叉搜索树
void inorder(TreeNode* root) {
if (root == NULL) {
return;
}
inorder(root->left);
printf("%d ", root->val);
inorder(root->right);
}
int main() {
// 初始化为空的二叉搜索树
TreeNode* root = NULL;
// 输入10个不同整数
int n = 10;
int nums[] = {8, 4, 12, 2, 6, 10, 14, 1, 3, 5};
// 依次插入到二叉搜索树中
for (int i = 0; i < n; i++) {
root = insert(root, nums[i]);
}
// 对二叉搜索树进行中序遍历,输出结果
inorder(root);
return 0;
}
```
输出结果为:1 2 3 4 5 6 8 10 12 14,说明二叉搜索树构建正确。
相关推荐
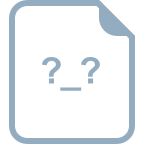
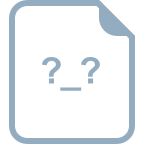











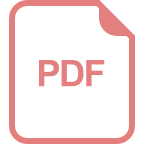
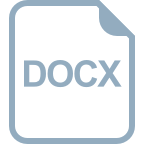
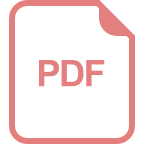