c语言输入10个不同整数,依次插入到一颗初始为空的二叉排序树中,并对其进行中序遍历,以验证树的正确性的代码
时间: 2023-12-03 08:42:43 浏览: 42
```
#include <stdio.h>
#include <stdlib.h>
// 二叉树结构体
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 插入节点
TreeNode* insert(TreeNode *root, int val) {
if (root == NULL) { // 根节点为空,新建节点
TreeNode *node = (TreeNode*) malloc(sizeof(TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
if (val < root->val) { // 插入到左子树
root->left = insert(root->left, val);
} else { // 插入到右子树
root->right = insert(root->right, val);
}
return root;
}
// 中序遍历
void inorderTraversal(TreeNode *root) {
if (root == NULL) {
return;
}
inorderTraversal(root->left);
printf("%d ", root->val);
inorderTraversal(root->right);
}
int main() {
TreeNode *root = NULL; // 初始化为空树
int num[10];
printf("请输入10个不同的整数:\n");
for (int i = 0; i < 10; i++) {
scanf("%d", &num[i]);
root = insert(root, num[i]); // 插入节点
}
printf("中序遍历结果为:\n");
inorderTraversal(root); // 中序遍历
printf("\n");
return 0;
}
```
注意:该代码假设输入的10个数不相同,如果有相同的数,会导致插入失败。可以在 `insert` 函数中添加判断来解决这个问题。
相关推荐
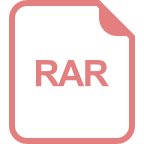
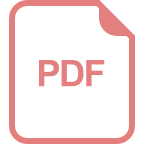













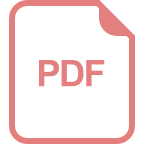
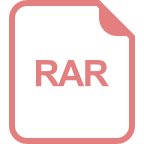