用Opencv编写一个图像梯度Laplace算法
时间: 2024-05-05 22:21:01 浏览: 98
好的,以下是使用OpenCV编写图像梯度Laplace算法的代码示例:
```python
import cv2
# 读取图像
img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
# 定义Laplace算子
laplacian_kernel = [[0, 1, 0], [1, -4, 1], [0, 1, 0]]
# 执行卷积操作
laplace_img = cv2.filter2D(img, -1, kernel=laplacian_kernel)
# 显示结果
cv2.imshow('Laplace Image', laplace_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
首先,使用`cv2.imread`函数读取图像,并将其转换为灰度图像。然后,定义Laplace算子,它是一个3x3的矩阵。在本例中,我们使用常见的Laplace算子,其中中心像素为-4,周围像素为1。最后,使用`cv2.filter2D`函数执行卷积操作,并将结果显示出来。
请注意,这只是一个简单的示例,可以根据实际需求进行修改和调整。
相关问题
针对输入的灰度图像,分别采用sobel、laplace或其他算法对图像进行边缘检测。(要求用C或C++语言实现)
以下是C++语言实现Sobel算子和Laplace算子进行边缘检测的示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取图像
Mat image = imread("lena.jpg", IMREAD_GRAYSCALE);
// 判断图像是否读取成功
if (image.empty())
{
cout << "Could not read the image!" << endl;
return -1;
}
// Sobel算子进行边缘检测
Mat sobel_x, sobel_y, sobel;
Sobel(image, sobel_x, CV_16S, 1, 0);
Sobel(image, sobel_y, CV_16S, 0, 1);
convertScaleAbs(sobel_x, sobel_x);
convertScaleAbs(sobel_y, sobel_y);
addWeighted(sobel_x, 0.5, sobel_y, 0.5, 0, sobel);
// Laplace算子进行边缘检测
Mat laplace;
Laplacian(image, laplace, CV_16S);
convertScaleAbs(laplace, laplace);
// 显示结果
imshow("Original Image", image);
imshow("Sobel Edge Detection", sobel);
imshow("Laplace Edge Detection", laplace);
waitKey(0);
return 0;
}
```
在该示例代码中,我们使用了OpenCV库中的Sobel()和Laplacian()函数来实现Sobel算子和Laplace算子进行边缘检测。其中,Sobel()函数用于计算图像的水平和垂直梯度,进而得到梯度幅值和方向,从而实现边缘检测;而Laplacian()函数则是直接计算图像的二阶导数,也可用于边缘检测。
注意:在实际使用中,我们需要根据具体的应用场景和要求选择合适的边缘检测算法,并进行参数调整和优化。
阅读全文
相关推荐
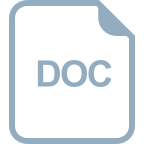
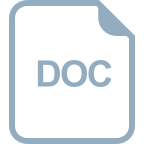
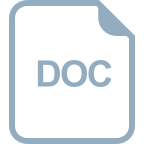
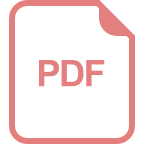
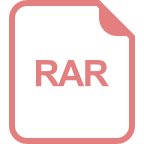
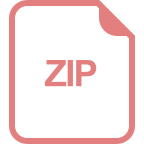
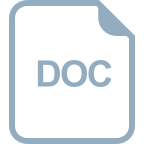
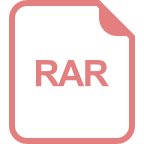
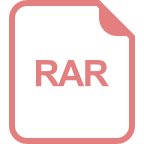
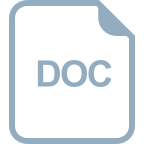
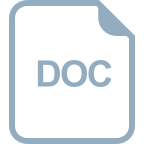
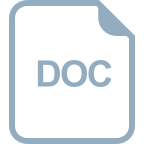
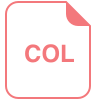
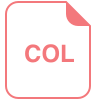
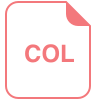
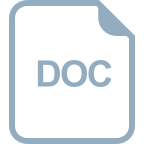
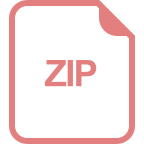