要求使用C语言编写程序,该程序有以下要求,要求使用三个进程完成三个任务,并且进程一和进程二之间的数据传送必须通过管道一进行,进程二和进程三之间的数据传送必须通过管道二进行。其中,data.txt文件中存放的是10个整数,result.txt一开始并不存在。首先进程一读取data.txt中的所有数据,并写入管道一中,进程二从管道一中取出数据并将所有的数据相加,并将这个结果写入管道二。进程三从管道二中取出结果,并将其输出成为一个名为result.txt的文件。请给出具体的C语言代码
时间: 2024-03-25 22:41:57 浏览: 24
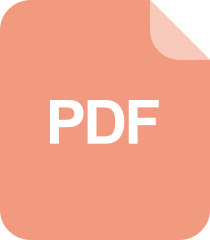
C语言编写linux下的守护进程
好的,以下是符合要求的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/wait.h>
#define PIPE1_READ 0
#define PIPE1_WRITE 1
#define PIPE2_READ 2
#define PIPE2_WRITE 3
int main() {
int pipe1[2], pipe2[2];
pid_t pid1, pid2;
int sum = 0;
char buf[256];
if (pipe(pipe1) < 0 || pipe(pipe2) < 0) {
perror("pipe error");
exit(1);
}
if ((pid1 = fork()) < 0) {
perror("fork error");
exit(1);
} else if (pid1 == 0) {
close(pipe1[PIPE_READ]);
int fd = open("data.txt", O_RDONLY);
if (fd < 0) {
perror("open file error");
exit(1);
}
while (read(fd, buf, sizeof(buf)) > 0) {
write(pipe1[PIPE_WRITE], buf, sizeof(buf));
}
close(fd);
close(pipe1[PIPE_WRITE]);
exit(0);
}
if ((pid2 = fork()) < 0) {
perror("fork error");
exit(1);
} else if (pid2 == 0) {
close(pipe1[PIPE_WRITE]);
close(pipe2[PIPE_READ]);
while (read(pipe1[PIPE_READ], buf, sizeof(buf)) > 0) {
sum += atoi(buf);
}
sprintf(buf, "%d", sum);
write(pipe2[PIPE_WRITE], buf, sizeof(buf));
close(pipe1[PIPE_READ]);
close(pipe2[PIPE_WRITE]);
exit(0);
}
close(pipe1[PIPE_WRITE]);
close(pipe2[PIPE_WRITE]);
int fd = open("result.txt", O_CREAT | O_WRONLY, 0644);
if (fd < 0) {
perror("open file error");
exit(1);
}
while (read(pipe2[PIPE_READ], buf, sizeof(buf)) > 0) {
write(fd, buf, sizeof(buf));
}
close(fd);
close(pipe1[PIPE_READ]);
close(pipe2[PIPE_READ]);
waitpid(pid1, NULL, 0);
waitpid(pid2, NULL, 0);
return 0;
}
```
其中,进程一读取data.txt中的所有数据,并写入管道一中,进程二从管道一中取出数据并将所有的数据相加,并将这个结果写入管道二。进程三从管道二中取出结果,并将其输出成为一个名为result.txt的文件。
阅读全文
相关推荐
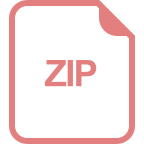
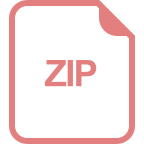


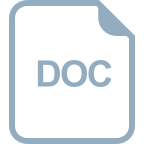








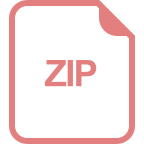
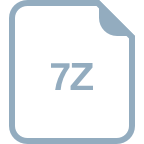