用C语言编写父进程fork三个子进程: 一个调用ps命令 一个调用自定义应用程序 一个调用会出现段错误的程序 父进程回收三个子进程(waitpid),并且打印三个子进程的退出状态
时间: 2024-02-20 18:02:20 浏览: 70
好的,我明白了。下面是代码实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main()
{
pid_t pid1, pid2, pid3;
int status1, status2, status3;
// fork第一个子进程,调用ps命令
pid1 = fork();
if (pid1 == 0) {
execl("/bin/ps", "ps", NULL);
}
else if (pid1 < 0) {
printf("fork failed\n");
exit(1);
}
// fork第二个子进程,调用自定义应用程序
pid2 = fork();
if (pid2 == 0) {
execl("./myapp", "myapp", NULL);
}
else if (pid2 < 0) {
printf("fork failed\n");
exit(1);
}
// fork第三个子进程,调用会出现段错误的程序
pid3 = fork();
if (pid3 == 0) {
char *p = NULL;
*p = 1;
}
else if (pid3 < 0) {
printf("fork failed\n");
exit(1);
}
// 等待三个子进程退出
waitpid(pid1, &status1, 0);
waitpid(pid2, &status2, 0);
waitpid(pid3, &status3, 0);
// 打印三个子进程的退出状态
printf("pid1: %d, status: %d\n", pid1, status1);
printf("pid2: %d, status: %d\n", pid2, status2);
printf("pid3: %d, status: %d\n", pid3, status3);
return 0;
}
```
注意,第三个子进程调用了会出现段错误的程序,所以它会在调用时崩溃。这也是我们故意设置的,为了测试父进程能否正确地回收子进程。
阅读全文
相关推荐
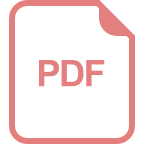
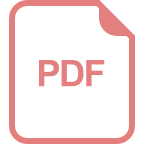
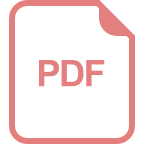











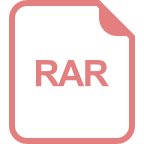
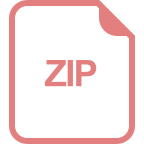