java实现四班三运转,每天早晨8:30、下午:16:30、晚上00:30进行换班,每个班按工作四天休一天再工作两天休一天规律进行排班,具体代码示例
时间: 2024-09-26 21:18:42 浏览: 10
在Java中实现这样的四班三运转排班规则,可以创建一个班次类`Shift`和一个调度类`Scheduling`来进行描述。下面是一个简单的代码示例:
首先,创建一个表示班次的Shift类:
```java
import java.time.LocalDateTime;
public class Shift {
private LocalDateTime startTime;
private String shiftType; // "早班"、"中班"或"夜班"
public Shift(String type, LocalDateTime time) {
this.startTime = time;
this.shiftType = type;
}
public LocalDateTime getStartTime() {
return startTime;
}
public String getShiftType() {
return shiftType;
}
@Override
public String toString() {
return "Shift{" +
"startTime=" + startTime +
", shiftType='" + shiftType + '\'' +
'}';
}
}
```
接着,创建一个调度类`Scheduling`来维护轮班和休假情况:
```java
import java.util.ArrayList;
import java.util.List;
import java.time.LocalDate;
public class Scheduling {
private List<Shift> schedule;
private LocalDate currentDate;
public Scheduling() {
this.schedule = new ArrayList<>();
currentDate = LocalDate.now(); // 初始化当前日期
}
public void addShift(Shift shift) {
schedule.add(shift);
}
public boolean isWorkingDay(LocalDate date) {
// 判断给定日期是否为工作日
// 这里简化处理,假设每月的第一天和最后一天是休息日,其余为工作日
return !date.equals(currentDate.plusDays(date.lengthOfMonth()))
&& !date.equals(currentDate.minusDays(date.lengthOfMonth()));
}
public void nextDay() {
currentDate = currentDate.plusDays(1);
}
public List<Shift> getNextFourDays() {
List<Shift> result = new ArrayList<>();
while (schedule.size() > 0 && isWorkingDay(currentDate)) {
result.add(schedule.remove(0));
nextDay();
}
return result;
}
public static void main(String[] args) {
Scheduling scheduling = new Scheduling();
// 添加初始班次(例如:早班8:30)
Shift morningShift = new Shift("早班", LocalDateTime.of(LocalDate.now(), LocalTime.of(8, 30)));
scheduling.addShift(morningShift);
// 模拟循环添加后续班次和休假
// ... (根据需求编写这部分逻辑)
// 获取并打印接下来四天的工作安排
List<Shift> fourDaysSchedule = scheduling.getNextFourDays();
for (Shift shift : fourDaysSchedule) {
System.out.println(shift);
}
}
}
```
这个例子展示了如何基本地管理四班三运转的排班,实际应用中可能需要更复杂的逻辑来处理每个月的特殊日期以及员工的具体假期安排。
相关推荐





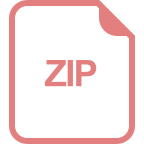
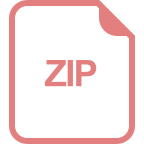
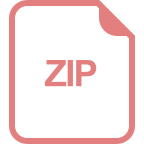
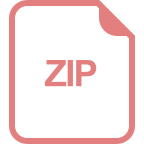
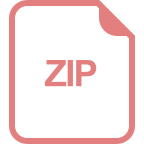
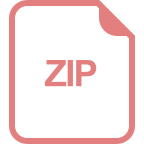
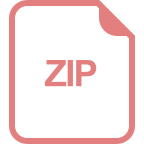
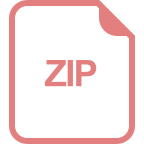
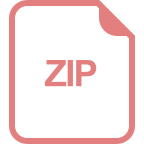
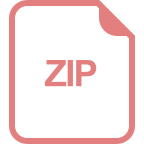
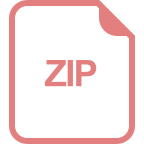