用栈解决迷宫问题c语言代码
时间: 2023-10-11 16:08:03 浏览: 61
根据引用所提供的内容,下面是用C语言编写的栈解决迷宫问题的代码:
```c
#include <stdio.h>
#define MAXSIZE 100
typedef struct {
int row;
int col;
} Position;
typedef struct {
Position data[MAXSIZE];
int top;
} Stack;
void initStack(Stack *s) {
s->top = -1;
}
void push(Stack *s, Position p) {
if (s->top == MAXSIZE - 1) {
printf("Stack is full.\n");
return;
}
s->top++;
s->data[s->top] = p;
}
void pop(Stack *s) {
if (s->top == -1) {
printf("Stack is empty.\n");
return;
}
s->top--;
}
int isEmpty(Stack s) {
return s.top == -1;
}
Position getTop(Stack s) {
return s.data[s.top];
}
int main() {
int mg[5][5] = {{0, 1, 0, 0, 0},
{0, 1, 0, 1, 0},
{0, 0, 0, 0, 0},
{0, 1, 1, 1, 0},
{0, 0, 0, 1, 0}};
Stack s;
initStack(&s);
Position start = {0, 0};
push(&s, start);
mg[start.row][start.col] = -1;
while (!isEmpty(s)) {
Position curPos = getTop(s);
if (curPos.row == 4 && curPos.col == 4) {
printf("Find the path!\n");
break;
}
int i, newRow, newCol;
for (i = 0; i < 4; i++) {
switch (i) {
case 0:
newRow = curPos.row - 1;
newCol = curPos.col;
break;
case 1:
newRow = curPos.row;
newCol = curPos.col + 1;
break;
case 2:
newRow = curPos.row + 1;
newCol = curPos.col;
break;
case 3:
newRow = curPos.row;
newCol = curPos.col - 1;
break;
}
if (newRow >= 0 && newRow < 5 && newCol >= 0 && newCol < 5 && mg[newRow][newCol] == 0) {
Position newPos = {newRow, newCol};
push(&s, newPos);
mg[newRow][newCol] = -1;
break;
}
}
if (i == 4) {
pop(&s);
}
}
if (isEmpty(s)) {
printf("No path found.\n");
}
return 0;
}
```
相关推荐
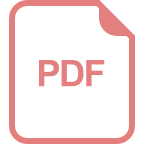












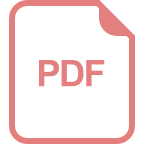
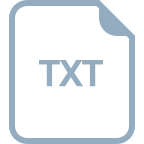