python 如何查找某个元素在 列表中的位置
时间: 2024-10-11 18:05:13 浏览: 66
在Python中,你可以使用`index()`方法来查找列表中特定元素的位置。这个方法会返回第一个找到的指定元素的索引,如果元素不存在于列表中,则会抛出`ValueError`异常。以下是基本的使用方式:
```python
# 定义一个列表
fruits = ['apple', 'banana', 'cherry', 'date']
# 查找元素 'banana' 的位置
position = fruits.index('banana')
# 打印结果
print(f"The position of 'banana' is {position}") # 输出:1
# 如果元素不存在,尝试查找会抛出错误
try:
position = fruits.index('grape')
except ValueError:
print("Element not found in the list.")
```
如果不确定元素是否存在于列表中,你也可以选择使用`enumerate()`函数结合循环来找出元素的位置,这样即使找不到也不会报错:
```python
for i, fruit in enumerate(fruits):
if fruit == 'grape':
print(f"Element 'grape' found at position {i}")
break
else:
print("Element not found in the list.")
```
相关问题
python查找列表元素位置
Python中可以使用index()函数来查找列表中某个元素的位置。
例如,假设有一个列表a=[1,2,3,4,5],想要查找元素3的位置,可以使用以下代码:
```
a=[1,2,3,4,5]
index = a.index(3)
print(index)
```
输出结果为:
```
2
```
注意:如果列表中不存在要查找的元素,则会抛出ValueError异常。
python 查询某个元素在多级列表中的位置
在Python中,查询某个元素在多级(嵌套)列表中的位置可以使用递归函数或者迭代的方式。这里我们以递归为例来说明:
假设你有一个多级列表例如:
```python
nested_list = [[1, 2, 3], [4, [5, 6]], [7, 8, [9, 'elephant']]]
```
你可以定义一个递归函数`find_element_index`,这个函数接受目标元素和当前层级的列表作为输入,返回元素的位置:
```python
def find_element_index(element, nested_list, level=0):
for index, value in enumerate(nested_list):
if isinstance(value, list): # 如果值是列表,则继续递归查找
sub_index = find_element_index(element, value, level + 1)
if sub_index is not None:
return (level, sub_index) # 返回元素在多层列表中的完整索引
elif value == element: # 如果找到目标元素,返回当前层级和索引
return (level, index)
return None # 如果遍历完都没有找到,返回None
element_to_find = 'elephant'
position = find_element_index(element_to_find, nested_list)
if position:
print(f"元素 '{element_to_find}' 的位置是: {position}")
else:
print("元素未在列表中找到。")
```
阅读全文
相关推荐
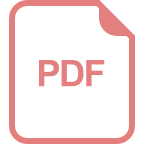
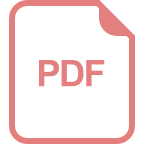
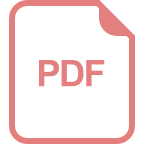













