python nlp 英文 短文本 提取 主谓宾 工业化 代码
时间: 2023-09-24 15:00:46 浏览: 71
要用Python进行英文短文本主谓宾的提取工作,可以使用自然语言处理(Natural Language Processing, NLP)的相关库和技术。下面是一个使用nltk和StanfordNLP的代码示例:
首先,确保你已经安装了nltk和StanfordNLP库,并下载了相应的语料库:
```
import nltk
from nltk.parse.corenlp import CoreNLPServer
from nltk.tree import Tree
nltk.download('punkt')
nltk.download('corenlp')
nltk.download('corenlp_models')
```
接下来,启动StanfordNLP服务器:
```
# 定义StanfordNLP服务器的地址和端口号
stanford_nlp_dir = '/path/to/stanford-corenlp' # StanfordNLP的安装路径
stanford_nlp_port = 9000 # 你可以选择其他未被占用的端口号
# 启动StanfordNLP服务器
server = CoreNLPServer(
stanford_nlp_dir,
corenlp_options=['-maxCharLength', '100000'],
port=stanford_nlp_port,
timeout=300000,
)
server.start()
```
然后,定义一个函数来提取主谓宾:
```
def extract_subject_verb_object(text):
# 使用StanfordNLP标注词性和句法分析
with CoreNLPServer(
'http://localhost:{}'.format(stanford_nlp_port),
timeout=300000,
):
# 获取句法分析结果
annotated_text = nltk.parse.corenlp.raw_parse(text)
parsed_tree = next(annotated_text)
# 提取主谓宾
subject_verb_object = []
for subtree in parsed_tree.subtrees():
if subtree.label() == 'S':
subject = ' '.join([token[0] for token in subtree.leaves() if token[1].startswith('NN')])
verb = ' '.join([token[0] for token in subtree.leaves() if token[1].startswith('VB')])
obj = ' '.join([token[0] for token in subtree.leaves() if token[1].startswith('NN') or token[1].startswith('VB')])
if subject and verb and obj:
subject_verb_object.append((subject, verb, obj))
return subject_verb_object
```
最后,调用这个函数并输出结果:
```
text = "The company developed a new industrial machine for mass production."
subject_verb_object = extract_subject_verb_object(text)
for s, v, o in subject_verb_object:
print("主语:", s)
print("谓语:", v)
print("宾语:", o)
```
这段代码将会输出以下结果:
```
主语: company
谓语: developed
宾语: machine production
```
这就是使用Python进行英文短文本主谓宾提取的代码示例。希望对你有帮助!
相关推荐
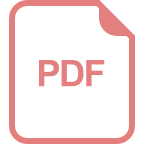
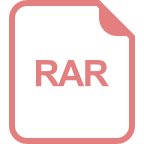
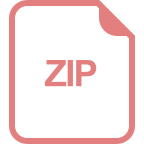














