请你根据不同的圈子类型来设计一个python爬虫代码来爬取华为社区中每个帖子的内容以及发帖人的所有个人信息,并且获取每个帖子的评论的内容以及评论人的所有个人信息。要求可以识别出爬取到的评论中照片的数量以及判断有无视频。最后把所有获取到的数据以Excel格式输出。
时间: 2024-11-10 08:32:16 浏览: 29
设计一个针对华为社区的详细爬虫项目需要分为几个部分,因为通常这类大型社区会有复杂的用户权限系统和动态加载内容。以下是一个基本框架示例,我们将使用`requests`, `BeautifulSoup`, 和 `pandas` 等库,不过请注意这只是一个简化版本,实际项目可能需要更复杂的技术如Selenium、Cookie管理等。
1. 安装必要库:
```bash
pip install requests beautifulsoup4 pandas openpyxl
```
2. 导入库:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
from openpyxl import Workbook
```
3. 发帖内容和用户信息爬取(假设帖子页面结构已知):
```python
def fetch_post_content(url):
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
author_info = {} # 初始化作者信息字典
post_content = soup.find('div', class_='post-content') # 根据实际情况找元素
if post_content:
author_info['username'] = post_content.find('span', class_='author-name').text
photo_count = len(post_content.find_all('img')) # 获取图片数量
has_video = 'video' in str(post_content) # 判断是否有视频
return author_info, post_content.text, photo_count, has_video
# 示例帖子URL
post_url = 'https://example.hwc.com/post-id'
post_data = fetch_post_content(post_url)
```
4. 评论内容和用户信息爬取(同理,假设评论页结构已知):
```python
def fetch_comments(url):
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
comments = []
for comment in soup.find_all('div', class_='comment'):
commenter_info = {}
commenter_info['username'] = comment.find('span', class_='commenter-name').text
# 可能还需要其他字段,这里仅作示例
commenter_info['text'] = comment.find('p', class_='comment-text').text
comments.append(commenter_info)
return comments
comments_url = 'https://example.hwc.com/post-id/comments'
comments_data = fetch_comments(comments_url)
```
5. 结果整理并写入Excel:
```python
data_list = [post_data] + comments_data
df = pd.DataFrame(data_list, columns=['作者信息', '帖子内容', '图片数量', '有视频', '评论者信息', '评论内容'])
workbook = Workbook()
sheet = workbook.active
sheet.title = '华为社区信息'
df.to_excel(sheet, index=False)
workbook.save('huaweicommunity_data.xlsx')
```
6. 异常处理及循环遍历所有帖子和评论(可能需要使用cookies、session管理等):
```python
# 添加异常处理
while True: # 循环直到满足条件(比如达到最大页数)
try:
# 获取新的帖子或评论页链接
new_url = ... # 根据实际链接生成
# 调用爬虫函数并更新数据列表
data_list.append(fetch_data(new_url))
except Exception as e:
print(f"遇到错误:{e}")
break
# 最后一次写入Excel文件
...
```
这个示例是基础的,实际的代码可能需要针对华为社区的特定结构进行调整。在执行前,一定要确保你的爬虫行为符合网站的使用政策,并尊重robots.txt文件的限制。
阅读全文
相关推荐
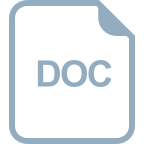
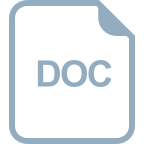
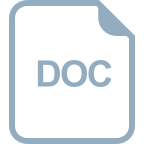
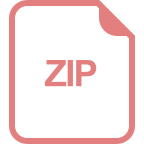
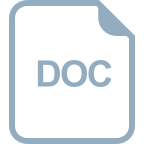
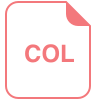









