program a division algorithm without division-instructions for 32-bit integer in C language
时间: 2023-12-10 09:40:56 浏览: 96
One possible way to implement a division algorithm without using division instructions in C language for 32-bit integers is to use repeated subtraction. Here's an example implementation:
```c
unsigned int divide(unsigned int dividend, unsigned int divisor) {
unsigned int quotient = 0;
while (dividend >= divisor) {
dividend -= divisor;
quotient++;
}
return quotient;
}
```
This function takes two unsigned integers, `dividend` and `divisor`, and returns their quotient as an unsigned integer. It repeatedly subtracts `divisor` from `dividend` until `dividend` becomes less than `divisor`. The number of times this subtraction operation is performed is the quotient.
Note that this algorithm does not handle division by zero. Also, since it uses repeated subtraction, it may be slow for large values of `dividend` and `divisor`. There are more efficient algorithms for division, but they typically require more complex implementation.
阅读全文
相关推荐


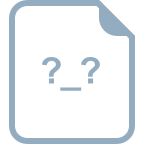











