program a 32-bit integer divide rapid algorithm without any float type in c langugage
时间: 2024-03-22 11:40:02 浏览: 11
Certainly, here's an implementation of a 32-bit integer division algorithm in C language without using any float types:
```
uint32_t divu32(uint32_t dividend, uint32_t divisor) {
uint32_t quotient = 0;
uint32_t remainder = 0;
for (int i = 31; i >= 0; i--) {
remainder <<= 1;
remainder |= (dividend >> i) & 1;
if (remainder >= divisor) {
remainder -= divisor;
quotient |= 1 << i;
}
}
return quotient;
}
```
This algorithm uses a simple bit-shifting and subtraction approach to compute the quotient of the division. The dividend is shifted one bit at a time, and the remainder is updated accordingly. If the remainder is greater than or equal to the divisor, then the divisor is subtracted from the remainder, and the corresponding bit in the quotient is set. This process is repeated until all bits in the dividend have been processed.
Note that this implementation assumes that the inputs are both unsigned 32-bit integers. Also, this implementation does not handle division by zero.
相关推荐
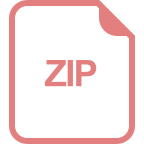
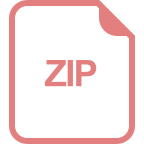
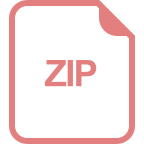















