使用flask实现修改密码功能
时间: 2023-07-18 10:05:58 浏览: 124
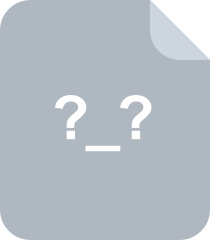
密码修改程序
首先,需要引入 Flask 和相关的模块:
```python
from flask import Flask, render_template, request, redirect, url_for, flash
from flask_sqlalchemy import SQLAlchemy
from werkzeug.security import generate_password_hash, check_password_hash
```
然后,需要创建 Flask 应用程序实例,并且设置数据库连接:
```python
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///users.db'
app.config['SECRET_KEY'] = 'your_secret_key'
db = SQLAlchemy(app)
```
接下来,定义用户数据模型:
```python
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(50), unique=True)
password = db.Column(db.String(100))
```
然后,定义修改密码的路由和视图函数:
```python
@app.route('/change_password', methods=['GET', 'POST'])
def change_password():
if request.method == 'POST':
current_password = request.form['current_password']
new_password = request.form['new_password']
confirm_password = request.form['confirm_password']
user = User.query.filter_by(username=session['username']).first()
if check_password_hash(user.password, current_password):
if new_password == confirm_password:
user.password = generate_password_hash(new_password)
db.session.commit()
flash('Password changed successfully!', 'success')
return redirect(url_for('profile'))
else:
flash('New password and confirm password do not match!', 'error')
else:
flash('Current password is incorrect!', 'error')
return render_template('change_password.html')
```
最后,在 HTML 模板文件中添加表单:
```html
{% extends 'base.html' %}
{% block content %}
<h1>Change Password</h1>
{% with messages = get_flashed_messages() %}
{% if messages %}
<ul class="messages">
{% for message in messages %}
<li class="{{ message[0] }}">{{ message[1] }}</li>
{% endfor %}
</ul>
{% endif %}
{% endwith %}
<form method="post">
<label for="current_password">Current Password:</label>
<input type="password" name="current_password" id="current_password" required>
<br>
<label for="new_password">New Password:</label>
<input type="password" name="new_password" id="new_password" required>
<br>
<label for="confirm_password">Confirm Password:</label>
<input type="password" name="confirm_password" id="confirm_password" required>
<br>
<input type="submit" value="Change Password">
</form>
{% endblock %}
```
这样就完成了使用 Flask 实现修改密码功能。需要注意的是,密码需要使用哈希函数进行加密,以保护用户的隐私。
阅读全文
相关推荐
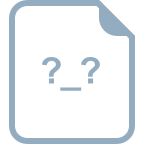


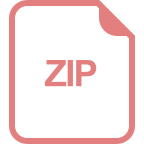
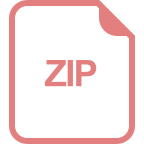
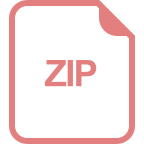
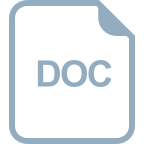
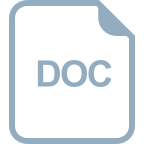
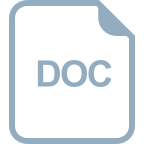
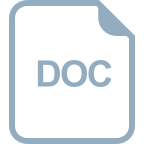
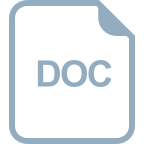
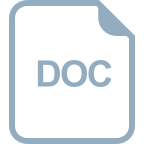
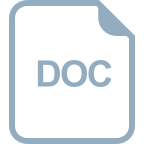
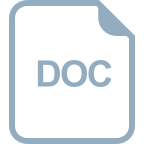
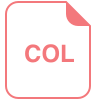

