contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE) img_bgr = cv2.cvtColor(imm, cv2.COLOR_GRAY2BGR) # 绘制边缘 # imm = cv2.drawContours(img_bgr, contours, -1, (0, 0, 255), 1) # 遍历轮廓 rects = [] for contour in contours: rect = cv2.minAreaRect(contour) rects.append(rect) box = cv2.boxPoints(rect) box = np.int0(box)在此代码中box[][]两个括号里分别是什么
时间: 2024-04-01 19:34:52 浏览: 65
在这段代码中,`box` 也是一个包含矩形四个角点坐标的 numpy 数组。`boxPoints()` 函数接收一个旋转矩形,返回一个包含四个点坐标的数组。
`box` 数组的两个方括号分别代表行和列。每个方括号内的数字代表该元素在数组中的位置。例如,`box[0][0]` 表示数组的第一行第一列元素,即第一个点的 x 坐标,`box[1][2]` 表示数组的第二行第三列元素,即第二个点的 y 坐标。因为 `box` 是一个二维数组,所以需要使用两个方括号来进行索引。
相关问题
请使用cv2.findContours函数写一个识别黑色矩形的python程序
好的,这里是一个使用cv2.findContours函数识别黑色矩形的Python程序:
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 转为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 二值化
ret, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 查找轮廓
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 遍历轮廓
for contour in contours:
# 计算轮廓周长
perimeter = cv2.arcLength(contour, True)
# 近似轮廓
approx = cv2.approxPolyDP(contour, 0.04 * perimeter, True)
# 如果轮廓是矩形
if len(approx) == 4:
# 确保矩形是黑色的
x, y, w, h = cv2.boundingRect(contour)
roi = image[y:y+h, x:x+w]
hsv = cv2.cvtColor(roi, cv2.COLOR_BGR2HSV)
lower_black = np.array([0, 0, 0])
upper_black = np.array([180, 255, 30])
mask = cv2.inRange(hsv, lower_black, upper_black)
if cv2.countNonZero(mask) == 0:
# 在图像上绘制矩形
cv2.drawContours(image, [contour], -1, (0, 255, 0), 2)
# 显示图像
cv2.imshow('image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个程序中,我们首先读取了一张图像,并将其转化为灰度图像。然后使用cv2.threshold函数将其二值化,并使用cv2.findContours函数查找轮廓。接下来,我们遍历每个轮廓,并使用cv2.approxPolyDP函数将其近似为一个多边形。如果近似后的多边形有4个顶点,则我们认为它是一个矩形。然后,我们检查矩形是否是黑色的,如果是,则在图像上绘制矩形。最后,我们显示结果图像。
cv2.findContours not enough value
The `cv2.findContours` function requires at least two arguments: the input image and the contour retrieval mode. If you are getting an error message saying "not enough value", it is likely because you are not providing enough arguments to the function.
Here is an example of how to use the `cv2.findContours` function:
``` python
import cv2
# Load an image
img = cv2.imread('image.png')
# Convert the image to grayscale
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Threshold the image to create a binary image
thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY)[1]
# Find contours in the binary image
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
```
In this example, `thresh` is the binary image that we want to find contours in. The second argument to `cv2.findContours` is the contour retrieval mode, which specifies how the contours should be retrieved. In this case, we are using `cv2.RETR_TREE`, which retrieves all of the contours and reconstructs a full hierarchy of nested contours. The third argument is the contour approximation method, which specifies how the contours should be approximated. In this case, we are using `cv2.CHAIN_APPROX_SIMPLE`, which compresses horizontal, vertical, and diagonal segments and leaves only their end points.
If you are still having trouble with the `cv2.findContours` function, please provide more information and code so that I can better understand the issue.
阅读全文
相关推荐
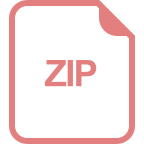
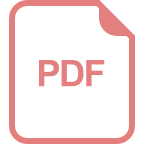
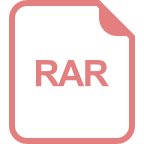














