c语言超市管理系统代码
时间: 2023-12-26 18:28:37 浏览: 34
根据提供的引用内容,以下是一个简单的C语言超市管理系统的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_PRODUCTS 100
struct Product {
int id;
char name[50];
float cost;
float price;
int stock;
};
struct Product products[MAX_PRODUCTS];
int numProducts = 0;
void addProduct() {
struct Product newProduct;
printf("Enter product ID: ");
scanf("%d", &newProduct.id);
printf("Enter product name: ");
scanf("%s", newProduct.name);
printf("Enter product cost: ");
scanf("%f", &newProduct.cost);
printf("Enter product price: ");
scanf("%f", &newProduct.price);
printf("Enter product stock: ");
scanf("%d", &newProduct.stock);
products[numProducts] = newProduct;
numProducts++;
printf("Product added successfully!\n");
}
void displayProducts() {
printf("Product ID\tProduct Name\tCost\tPrice\tStock\n");
for (int i = 0; i < numProducts; i++) {
printf("%d\t\t%s\t\t%.2f\t%.2f\t%d\n", products[i].id, products[i].name, products[i].cost, products[i].price, products[i].stock);
}
}
void purchaseProduct() {
int productId, quantity;
printf("Enter product ID: ");
scanf("%d", &productId);
printf("Enter quantity: ");
scanf("%d", &quantity);
for (int i = 0; i < numProducts; i++) {
if (products[i].id == productId) {
if (products[i].stock >= quantity) {
float totalCost = products[i].cost * quantity;
float totalPrice = products[i].price * quantity;
products[i].stock -= quantity;
printf("Total cost: %.2f\n", totalCost);
printf("Total price: %.2f\n", totalPrice);
return;
} else {
printf("Insufficient stock!\n");
return;
}
}
}
printf("Product not found!\n");
}
void menu() {
int choice;
do {
printf("\n-- Supermarket Management System --\n");
printf("1. Add product\n");
printf("2. Display products\n");
printf("3. Purchase product\n");
printf("4. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
addProduct();
break;
case 2:
displayProducts();
break;
case 3:
purchaseProduct();
break;
case 4:
printf("Exiting...\n");
break;
default:
printf("Invalid choice! Please try again.\n");
break;
}
} while (choice != 4);
}
int main() {
menu();
return 0;
}
```
相关推荐
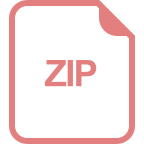
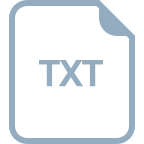














