C语言超市管理系统
时间: 2023-07-09 17:04:19 浏览: 169
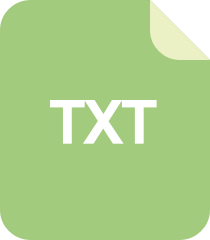
超市管理系统C源代码

好的,超市管理系统可以分为前台收银和后台管理两部分。下面是一个简单的C语言实现的超市管理系统的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义商品结构体
typedef struct {
char name[20];
float price;
int num;
} Goods;
// 初始化商品库存
Goods goods[100] = {
{"可乐", 2.5, 100},
{"薯片", 3.0, 80},
{"饼干", 4.0, 70},
{"方便面", 5.0, 90},
{"牛奶", 3.5, 120}
};
// 显示商品列表
void showGoods() {
printf("商品列表:\n");
printf("----------------------------------------\n");
printf("%-10s%-10s%-10s\n", "名称", "价格", "库存");
for (int i = 0; i < 5; i++) {
printf("%-10s%-10.2f%-10d\n", goods[i].name, goods[i].price, goods[i].num);
}
printf("----------------------------------------\n");
}
// 购物
void shopping() {
float totalMoney = 0;
char name[20];
int num;
while (1) {
showGoods();
printf("请输入商品名称和购买数量,以空格隔开(输入exit退出购物):\n");
scanf("%s", name);
if (strcmp(name, "exit") == 0) {
break;
}
scanf("%d", &num);
for (int i = 0; i < 5; i++) {
if (strcmp(name, goods[i].name) == 0) {
if (goods[i].num < num) {
printf("库存不足!\n");
} else {
goods[i].num -= num;
totalMoney += goods[i].price * num;
}
break;
}
if (i == 4) {
printf("商品不存在!\n");
}
}
}
printf("总计金额为:%.2f元\n", totalMoney);
}
int main() {
printf("欢迎使用超市管理系统!\n");
while (1) {
printf("请选择您要进行的操作(1.购物 2.退出系统):\n");
int choice;
scanf("%d", &choice);
switch (choice) {
case 1:
shopping();
break;
case 2:
printf("感谢使用超市管理系统,再见!\n");
exit(0);
default:
printf("输入有误,请重新选择!\n");
}
}
return 0;
}
```
以上代码实现了一个简单的超市管理系统,包括商品列表展示、购物和退出系统等功能。可以根据需求进行修改或扩展。
阅读全文
相关推荐
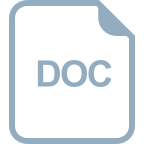
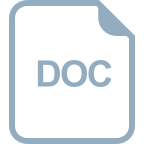
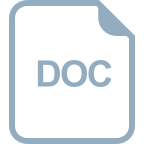
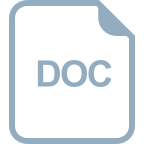
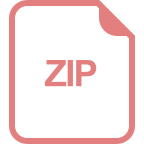
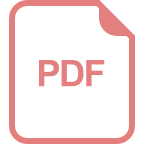
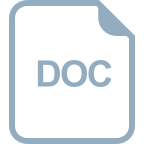
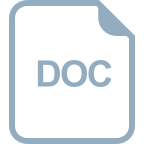
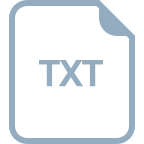
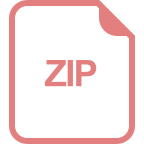
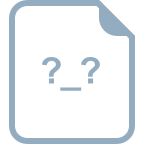
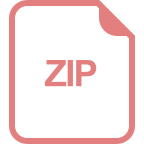

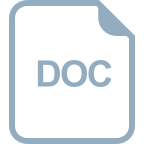
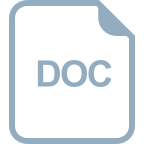
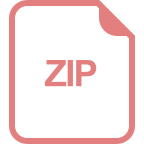