pytho建立一个csv文件
时间: 2024-01-21 12:04:07 浏览: 21
可以使用Python中的csv模块来创建一个csv文件。以下是一个简单的示例:
```python
import csv
# 创建csv文件并写入数据
with open('example.csv', mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Age', 'Gender'])
writer.writerow(['John', '25', 'Male'])
writer.writerow(['Jane', '30', 'Female'])
```
这将创建一个名为example.csv的文件,并将以下内容写入其中:
```
Name,Age,Gender
John,25,Male
Jane,30,Female
```
你可以根据需要修改数据和文件名。
相关问题
micpython怎么建立一个csv文件
要在Micropython中创建CSV文件,您需要使用文件系统模块来创建和操作文件。以下是一个示例代码,可以在Micropython中创建一个新的CSV文件并将数据写入其中:
```python
import os
filename = 'data.csv'
# check if the file already exists
if filename in os.listdir():
# if the file exists, delete it
os.remove(filename)
# create a new file and open it for writing
with open(filename, 'w') as file:
# write the header row
file.write('Name, Age, Gender\n')
# write some data rows
file.write('John, 25, M\n')
file.write('Jane, 30, F\n')
file.write('Bob, 40, M\n')
print('CSV file created successfully.')
```
该代码首先检查是否存在名为“data.csv”的文件,如果存在,则将其删除。然后,它创建一个新的文件并将其打开以进行写入。使用write()函数,它向文件中写入标题行,然后写入一些数据行。最后,它关闭文件并打印一个成功的消息。
请注意,您需要将代码中的文件名更改为您想要使用的名称,并且您可以根据需要更改标题行和数据行。
如何在python中建立打开一个csv文件
您可以使用Python内置的csv模块来打开和读取CSV文件。下面是一个简单的代码示例:
```python
import csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
```
在这个示例中,我们打开名为“example.csv”的文件,并使用csv.reader()函数将其读取为一个reader对象。然后,我们可以使用一个for循环遍历每一行,并将其打印出来。请确保将文件名替换为您自己的CSV文件名,以及使用正确的文件路径(如果需要)。
相关推荐
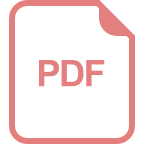
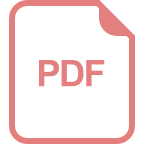
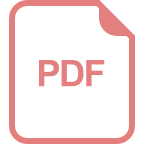
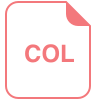
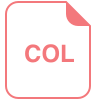
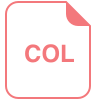
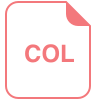








