使用python将dox文件的图片读取出来并放入新的dox文件中的指定位置
时间: 2024-05-06 08:22:16 浏览: 185
由于没有具体的dox文件和图片,以下是一个示例代码,演示如何将一个图片插入到另一个docx文件中的指定位置。
```python
from docx import Document
from docx.shared import Inches
# 打开原始docx文件
doc = Document('original.docx')
# 找到要插入图片的段落
para = doc.paragraphs[1]
# 插入图片
img_path = 'image.jpg'
para.add_run().add_picture(img_path, width=Inches(2))
# 保存新的docx文件
doc.save('new.docx')
```
在上面的示例代码中,我们打开了一个docx文件,找到要插入图片的段落,然后使用`add_picture()`方法将图片插入到段落中。最后,我们保存新的docx文件。注意,我们可以设置图片的宽度和高度,这里我们使用`Inches()`函数指定了宽度为2英寸。
相关问题
使用python将dox文件的图片取出来
有几种方法可以从docx文件中提取图片,以下是其中两种方法:
方法一:使用python-docx库
```python
import docx
# 打开docx文件
doc = docx.Document('example.docx')
# 遍历所有段落并提取图片
for para in doc.paragraphs:
for run in para.runs:
if run._element.tag.endswith('}rPr'):
for child in run._element:
if child.tag.endswith('}drawing'):
for grandchild in child:
if grandchild.tag.endswith('}graphic'):
for greatgrandchild in grandchild:
if greatgrandchild.tag.endswith('}graphicData'):
for item in greatgrandchild:
if item.tag.endswith('}pic'):
for i in item:
if i.tag.endswith('}blipFill'):
for j in i:
if j.tag.endswith('}blip'):
# 提取图片
img_data = j.attrib['{http://schemas.openxmlformats.org/officeDocument/2006/relationships}embed']
img_name = 'image_' + img_data.split('rId')[-1] + '.jpeg'
with open(img_name, 'wb') as f:
f.write(doc.part.related_parts[img_data].blob)
```
方法二:使用python unzip库
```python
import os
import zipfile
# 打开docx文件
docx_file = 'example.docx'
zip_docx = zipfile.ZipFile(docx_file)
# 遍历所有文件并提取图片
for file in zip_docx.namelist():
if file.endswith(('.jpeg', '.jpg', '.png', '.bmp')):
img_name = os.path.basename(file)
img_data = zip_docx.read(file)
with open(img_name, 'wb') as f:
f.write(img_data)
```
使用python将dox文件的内容写入新的dox文件中
以下是一个示例代码,可以将一个名为“old.docx”的Word文档中的内容复制到一个名为“new.docx”的新文档中。
```python
import docx
# 打开旧文档
old_doc = docx.Document('old.docx')
# 创建新文档
new_doc = docx.Document()
# 复制旧文档中的段落到新文档中
for paragraph in old_doc.paragraphs:
new_doc.add_paragraph(paragraph.text)
# 保存新文档
new_doc.save('new.docx')
```
请注意,此代码仅复制了旧文档中的段落。如果你想要复制其他内容,例如表格、图片或样式,请查阅Python-docx文档以了解更多信息。
阅读全文
相关推荐

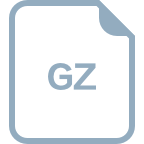







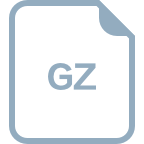

