使用3DES算法加密dox文件的c++代码
时间: 2024-10-15 15:13:03 浏览: 21
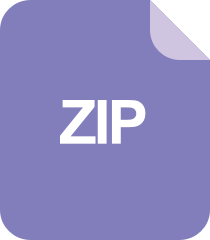
基于C++的网格实验库设计源码
在C++中使用3DES (Triple Data Encryption Standard) 加密文件,你需要包含相关的加密库(如openssl)并实现必要的加密步骤。以下是一个简单的示例,展示了如何使用OpenSSL库进行文件加密:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <openssl/evp.h>
std::string encryptFile(const std::string& inputFile, const std::string& outputFile, const std::string& key, const std::string& iv) {
// 加载私钥和初始向量
unsigned char* privKey = (unsigned char*)key.c_str();
unsigned char* ivBytes = (unsigned char*)iv.c_str();
// 创建一个EVP上下文
EVP_CIPHER_CTX ctx;
if (EVP_EncryptInit_ex(&ctx, EVP_3DES(), NULL, privKey, ivBytes) != 1) {
std::cerr << "Error initializing encryption context" << std::endl;
return "";
}
// 打开输入文件
std::ifstream in(inputFile, std::ios::binary);
if (!in) {
std::cerr << "Failed to open input file" << std::endl;
EVP_EncryptDestroy(&ctx);
return "";
}
// 打开输出文件用于二进制写入
std::ofstream out(outputFile, std::ios::binary);
if (!out) {
std::cerr << "Failed to create output file" << std::endl;
EVP_EncryptDestroy(&ctx);
in.close();
return "";
}
// 数据块大小
size_t blockSize = EVP_CIPHER_block_size(EVP_3DES());
// 编读数据
char buffer[blockSize];
while (in.read(buffer, blockSize)) {
if (in.gcount() == 0) break; // 如果读取结束
// 加密数据块
unsigned char encrypted[blockSize];
int len = EV_PKEY_length(privKey); // 3DES 密钥长度(8字节)
if (EVP_EncryptUpdate(&ctx, encrypted, &len, buffer, in.gcount()) <= 0) {
std::cerr << "Encryption failed" << std::endl;
break;
}
// 写入加密后的数据到输出文件
out.write(encrypted, len);
}
// 结束加密并写入最终的填充
unsigned char padding[blockSize];
memset(padding, 0, blockSize);
if (EVP_EncryptFinal_ex(&ctx, padding, &len) <= 0) {
std::cerr << "Encryption finalization error" << std::endl;
}
out.write(padding, len);
// 清理资源
in.close();
out.close();
EVP_EncryptDestroy(&ctx);
return "";
}
int main() {
std::string inputFile = "input.dox";
std::string outputFile = "output.enc";
std::string key = "your_key_here"; // 3DES 密钥
std::string iv = "your_iv_here"; // 初始化向量
if (encryptFile(inputFile, outputFile, key, iv).empty()) {
std::cerr << "Encryption failed" << std::endl;
} else {
std::cout << "File encrypted successfully." << std::endl;
}
return 0;
}
```
请注意,这个示例仅作为教学用途,实际使用时需确保妥善处理错误情况,并在生产环境中使用更安全的方式存储和传输密钥。
阅读全文
相关推荐
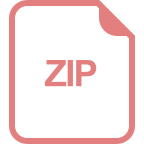
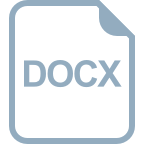
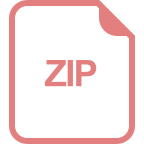
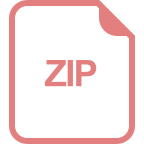
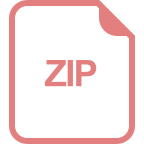
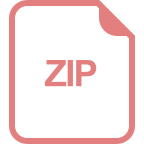
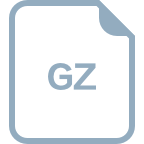
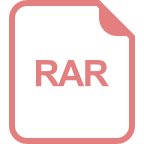
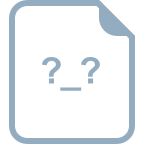






