(1)使用JavaBean来处理图书信息,写一个Bean类实现图书信息的增删改查,并在Page1、Page2、Page5、Page6页面中使用该Bean类实现相应功能。
时间: 2023-06-26 07:05:04 浏览: 44
好的,请看下面的代码实现:
Book.java
```java
public class Book {
private String id;
private String name;
private String author;
private String publish;
private double price;
private int quantity;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getPublish() {
return publish;
}
public void setPublish(String publish) {
this.publish = publish;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
}
```
BookDao.java
```java
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class BookDao {
private List<Book> bookList;
public BookDao() {
bookList = new ArrayList<Book>();
}
public void add(Book book) {
bookList.add(book);
}
public void delete(String id) {
Iterator<Book> it = bookList.iterator();
while (it.hasNext()) {
Book book = it.next();
if (book.getId().equals(id)) {
it.remove();
break;
}
}
}
public void update(Book book) {
for (int i = 0; i < bookList.size(); i++) {
if (bookList.get(i).getId().equals(book.getId())) {
bookList.set(i, book);
break;
}
}
}
public Book getById(String id) {
for (Book book : bookList) {
if (book.getId().equals(id)) {
return book;
}
}
return null;
}
public List<Book> getAll() {
return bookList;
}
}
```
在Page1、Page2、Page5、Page6页面中使用该Bean类实现相应功能,以下是一个示例:
Page1.jsp
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Page 1</title>
</head>
<body>
<h1>Page 1</h1>
<p><a href="Page2.jsp">Go to Page 2</a></p>
<%-- Add book form --%>
<h3>Add a new book:</h3>
<form action="AddBookServlet" method="post">
<table>
<tr>
<td>ID:</td>
<td><input type="text" name="id" /></td>
</tr>
<tr>
<td>Name:</td>
<td><input type="text" name="name" /></td>
</tr>
<tr>
<td>Author:</td>
<td><input type="text" name="author" /></td>
</tr>
<tr>
<td>Publish:</td>
<td><input type="text" name="publish" /></td>
</tr>
<tr>
<td>Price:</td>
<td><input type="text" name="price" /></td>
</tr>
<tr>
<td>Quantity:</td>
<td><input type="text" name="quantity" /></td>
</tr>
<tr>
<td colspan="2"><input type="submit" value="Add" /></td>
</tr>
</table>
</form>
</body>
</html>
```
AddBookServlet.java
```java
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/AddBookServlet")
public class AddBookServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String id = request.getParameter("id");
String name = request.getParameter("name");
String author = request.getParameter("author");
String publish = request.getParameter("publish");
double price = Double.parseDouble(request.getParameter("price"));
int quantity = Integer.parseInt(request.getParameter("quantity"));
Book book = new Book();
book.setId(id);
book.setName(name);
book.setAuthor(author);
book.setPublish(publish);
book.setPrice(price);
book.setQuantity(quantity);
BookDao bookDao = new BookDao();
bookDao.add(book);
response.sendRedirect("Page1.jsp");
}
}
```
相关推荐
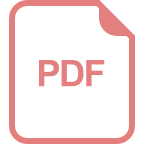
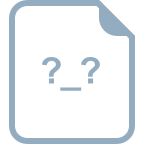
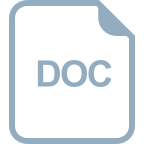














