用skimage.util.random_noise函数增加椒盐噪声如何实现
时间: 2023-05-17 08:07:16 浏览: 372
您可以使用以下代码来使用skimage.util.random_noise函数增加椒盐噪声:
```python
from skimage.util import random_noise
from skimage import io
# 读取图像
image = io.imread('image.jpg')
# 增加椒盐噪声
noisy_image = random_noise(image, mode='s&p', amount=0.05)
# 显示图像
io.imshow(noisy_image)
io.show()
```
在这个例子中,我们使用了skimage.util.random_noise函数来增加椒盐噪声。我们将mode参数设置为's&p',表示我们要增加椒盐噪声。amount参数表示噪声的数量,我们将其设置为0.05,表示噪声占图像的5%。最后,我们使用skimage的io模块来读取和显示图像。
相关问题
如何使用scikit-image库中的skimage.util.random_noise添加噪声
在scikit-image(skimage)库中,`skimage.util.random_noise`函数用于向图像添加随机噪声。以下是使用这个函数的基本步骤:
1. **安装scikit-image**:如果你还没有安装,首先确保已经安装了,如果没有,可以在命令行或终端中运行:
```powershell
pip install scikit-image
```
2. **导入所需模块**:
```python
from skimage import util
```
3. **加载或创建一个图像**:
```python
image = plt.imread('your_image_file.jpg') # 替换为你要处理的实际图片路径
```
4. **选择噪声类型**:`random_noise`函数支持多种类型的噪声,如高斯噪声、椒盐噪声等。例如,添加高斯噪声:
```python
noise_type = 'gaussian'
noisy_image = util.random_noise(image, mode=noise_type)
```
其他选项包括:
- `'salt'`: 椒盐噪声
- `'pepper'`: 黑白像素混合
- `'s&p'`: 同时包含椒盐和黑白像素
- `'speckle'`: 类似于高斯噪声但更细粒度
5. **查看结果**:
```python
plt.imshow(noisy_image, cmap='gray')
plt.show()
```
6. **保存噪声后的图像**(可选):
```python
plt.imsave('noisy_image.jpg', noisy_image)
```
注意,`mode`参数可以根据需要调整噪声的类型和强度。`util.random_noise`函数会返回一个新的图像数组,原图不会受到影响。
python中实现:(a) Load the image 'mandrill.jpg'. (b) Add salt&Pepper noise with different intensities (at least 3 kinds). (Tips: skimage.util.random_noise) (c) Perform smoothing linear filtering to smooth these noised images respectively. (Tips: ImageFilter.BLUR) (d) Display the images in the same figure with sub-figures. Add the corresponding title to each sub-figure.
和椒盐噪声到图像中。 (c) 使用中值滤波器去除噪声。
以下是实现该功能的Python代码:
```python
import cv2
import numpy as np
# Load the image
img = cv2.imread('mandrill.jpg')
# Add salt and pepper noise
noise = np.zeros(img.shape, np.uint8)
cv2.randu(noise, 0, 255)
salt = noise > 245
pepper = noise < 10
img[salt] = 255
img[pepper] = 0
# Apply median filter to remove noise
filtered_img = cv2.medianBlur(img, 5)
# Display the original and filtered images
cv2.imshow('Original Image', img)
cv2.imshow('Filtered Image', filtered_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码首先使用`cv2.imread()`函数加载了名为`mandrill.jpg`的图像。然后,使用`cv2.randu()`函数生成了与原始图像大小相同的随机噪声,并将其转换为二值图像,其中亮像素表示盐噪声,暗像素表示椒噪声。接下来,将盐噪声像素设置为255(白色),将椒噪声像素设置为0(黑色),从而将噪声添加到原始图像中。最后,使用`cv2.medianBlur()`函数对图像进行中值滤波,以去除噪声。最终,使用`cv2.imshow()`函数显示原始图像和过滤后的图像。
阅读全文
相关推荐






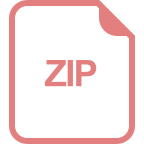
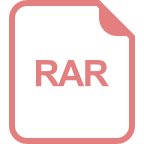
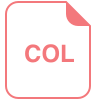




