python使用双线程模版
时间: 2023-09-16 16:05:59 浏览: 135
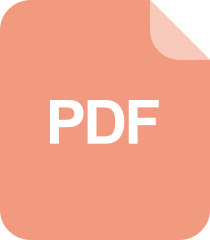
python实现多线程的两种方式
### 回答1:
你好!以下是使用 Python 进行双线程编程的示例模版:
```
import threading
def function_1():
# code for thread 1
def function_2():
# code for thread 2
# create threads
thread_1 = threading.Thread(target=function_1)
thread_2 = threading.Thread(target=function_2)
# start threads
thread_1.start()
thread_2.start()
# wait for threads to finish
thread_1.join()
thread_2.join()
```
在上面的代码中,我们创建了两个线程,分别执行 `function_1` 和 `function_2` 函数。然后启动这两个线程,最后使用 `join` 方法等待它们结束。
请注意,Python 的线程对象的 `start` 方法会启动一个新的线程,并在新线程中执行指定的函数。你也可以使用 `Thread` 类的构造函数来创建线程,然后使用 `run` 方法来启动线程。
```
import threading
def function_1():
# code for thread 1
def function_2():
# code for thread 2
# create threads
thread_1 = threading.Thread(target=function_1)
thread_2 = threading.Thread(target=function_2)
# start threads
thread_1.run()
thread_2.run()
# wait for threads to finish
thread_1.join()
thread_2.join()
```
希望这些信息对你有所帮助!
### 回答2:
Python提供了`threading`模块来实现多线程编程,以支持在程序中使用双线程模型。
双线程模型是指在一个程序中同时运行两个线程。可以使用Python的`threading.Thread`类来创建一个线程对象,并通过调用其`start()`方法来启动线程的执行。线程对象的`run()`方法定义了线程的执行逻辑,可以通过继承`Thread`类并覆写`run()`方法来自定义线程的行为。
双线程模型用于并发执行两个或多个任务,可以提高程序的运行效率和响应性。在Python中,可以使用`threading`模块来创建多个线程对象,并通过调用它们的`start()`方法使它们并发执行。线程之间可以共享数据,但需要注意数据安全问题,可以使用互斥锁(`threading.Lock`)来对共享资源进行保护。
Python的多线程编程依赖于全局解释器锁(GIL),即同一时间只能有一个线程执行Python字节码。因此,在进行CPU密集型任务时,多线程的效果可能不如预期。但是,对于I/O密集型任务,如网络请求、文件读写等,多线程可以显著提升程序的性能。
需要注意的是,使用多线程编程时要注意线程安全问题,避免出现数据竞争和死锁等问题。可以使用线程同步机制如锁、条件变量等来确保线程之间的正确协作。
总之,Python提供了`threading`模块来实现双线程模型,可以通过创建线程对象并调用其`start()`方法来实现多线程编程。多线程可以在某些情况下提高程序性能和响应性,但需要注意线程安全和GIL的限制。
### 回答3:
Python使用多线程的方式有很多,其中一种常用的方式是使用`threading`模块来创建和管理线程。
首先,需要导入`threading`模块:
```python
import threading
```
然后,可以定义一个函数作为线程的执行体,即线程要执行的任务:
```python
def thread_task():
# 线程的任务代码
print("This is a thread.")
```
接下来,可以创建一个线程对象,并将要执行的任务传递给线程对象的`target`参数:
```python
thread = threading.Thread(target=thread_task)
```
然后,可以通过调用线程对象的`start()`方法来启动线程:
```python
thread.start()
```
线程启动后,执行体函数`thread_task()`将会在新的线程中被调用并执行。
另外,也可以使用类继承`threading.Thread`的方式来创建线程类。首先,定义一个继承自`threading.Thread`的子类,并重写`run()`方法(该方法是线程的执行体):
```python
class MyThread(threading.Thread):
def run(self):
# 线程的任务代码
print("This is a thread.")
```
然后,创建线程对象并调用`start()`方法启动线程:
```python
thread = MyThread()
thread.start()
```
通过以上方式,可以在Python中创建和启动线程。但需要注意的是,由于GIL(全局解释器锁)的存在,Python中的多线程并不能真正实现并行执行,因为在任意给定的时间点,只有一个线程能够执行Python字节码。所以,对于需要实现真正并行执行的任务,可以考虑使用多进程、协程等方式来代替多线程。
阅读全文
相关推荐

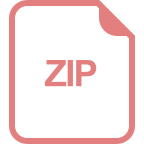
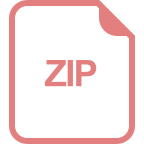
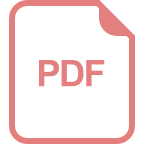
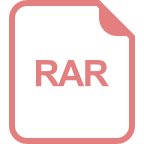
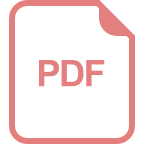
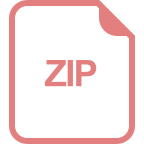
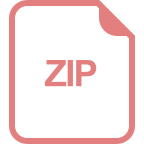
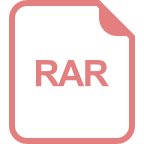
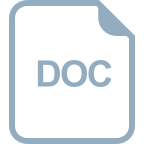
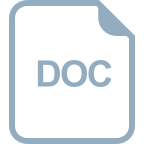
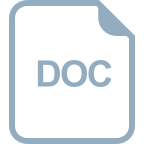