生成一个编写Python爬虫程序,爬取地址: https://www.luogu.com.cn/contest/68651#scoreboard中的比赛榜单, 读取《用户信息.xls文件》,如果用户信息中的账号不在榜单中则排名填-1,如果用户在榜单中则爬取用户的排名和题目通过情况(例:A题通过则填AC,否则留空)
时间: 2024-02-19 12:01:36 浏览: 25
好的,这是一个比较复杂的任务,需要用到 Python 的爬虫库和数据处理库。我会给您提供一个基本的思路,您可以根据自己的需要进行修改和完善。
首先,需要安装以下库:
- requests:用于发起 HTTP 请求获取网页内容;
- BeautifulSoup4:用于解析 HTML 和 XML 文档;
- pandas:用于读取和写入 Excel 文件。
您可以使用 pip 命令来安装这些库,比如:
```
pip install requests beautifulsoup4 pandas
```
然后,可以编写一个 Python 程序来实现爬取榜单和读取用户信息的功能。代码如下:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 爬取榜单
def get_scoreboard(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
scoreboard = []
for tr in soup.find_all('table')[0].find_all('tr')[1:]:
tds = tr.find_all('td')
scoreboard.append({
'rank': int(tds[0].text.strip()),
'username': tds[1].find_all('a')[0].text.strip(),
'score': int(tds[2].text.strip()),
'penalty': int(tds[3].text.strip()),
'solved': [td.text.strip() for td in tds[4:]]
})
return scoreboard
# 读取用户信息
def read_user_info(filename):
df = pd.read_excel(filename)
user_info = []
for i, row in df.iterrows():
user_info.append({
'username': row['用户名'],
'rank': -1,
'solved': row['已通过的题目'].split(',')
})
return user_info
# 匹配用户和榜单
def match_users(scoreboard, user_info):
for user in user_info:
found = False
for s in scoreboard:
if s['username'] == user['username']:
user['rank'] = s['rank']
for i, p in enumerate(user['solved']):
if p in s['solved']:
user['solved'][i] = 'AC'
else:
user['solved'][i] = ''
found = True
break
if not found:
user['rank'] = -1
user['solved'] = [''] * len(user['solved'])
# 输出结果到 Excel 文件
def output_to_excel(user_info, filename):
df = pd.DataFrame(user_info, columns=['username', 'rank', 'solved'])
df.to_excel(filename, index=False)
# 测试
if __name__ == '__main__':
scoreboard = get_scoreboard('https://www.luogu.com.cn/contest/68651#scoreboard')
user_info = read_user_info('用户信息.xls')
match_users(scoreboard, user_info)
output_to_excel(user_info, '结果.xls')
```
这个程序包含了三个主要的函数:
- `get_scoreboard`:用于爬取榜单,并返回一个包含排名、用户名、得分、罚时和已通过题目的列表;
- `read_user_info`:用于读取用户信息 Excel 文件,并返回一个包含用户名、排名和已通过题目的列表的列表;
- `match_users`:用于匹配用户和榜单,并将用户的排名和已通过题目更新到列表中。
在测试部分,我们先调用 `get_scoreboard` 和 `read_user_info` 函数来获取榜单和用户信息,然后调用 `match_users` 函数来匹配用户和榜单,最后调用 `output_to_excel` 函数将结果输出到 Excel 文件中。
这个程序可能还需要进行一些修改和调试,但是这个思路应该是可行的。如果您有任何问题或者需要进一步的帮助,请随时问我!
相关推荐
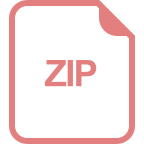
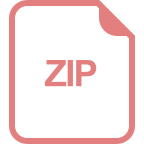






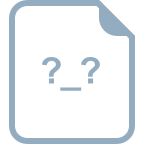
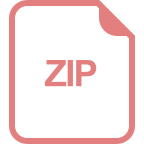
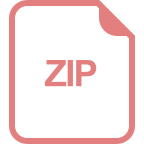
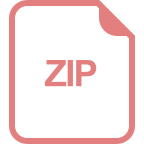
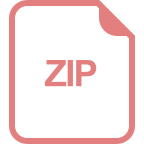
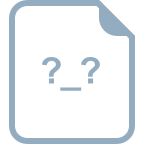