unity实现血条缓慢下降
时间: 2023-07-11 07:13:31 浏览: 149
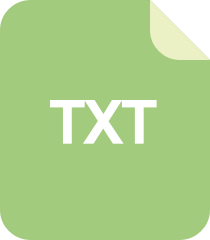
Unity-置顶OpenFileDialog文件选择框
要实现血条缓慢下降,可以使用定时器或协程来控制血条的减少速度。具体步骤如下:
1. 获取角色的当前血量值和最大血量值。
2. 使用定时器或协程来控制血条的减少速度。例如,每隔1秒钟减少1%的血量。
3. 在定时器或协程中更新血条的显示,即将当前血量值转换成血条的长度或百分比。
4. 当角色的血量值小于等于0时,停止定时器或协程,并执行相应的死亡逻辑。
下面是使用协程实现血条缓慢下降的示例代码:
```
using UnityEngine;
using UnityEngine.UI;
using System.Collections;
public class HealthBar : MonoBehaviour
{
public Image healthBar;
public float maxHealth = 100f;
public float currentHealth = 100f;
public float decreaseSpeed = 1f;
private Coroutine decreaseCoroutine;
void Start()
{
UpdateHealthBar();
decreaseCoroutine = StartCoroutine(DecreaseHealth());
}
IEnumerator DecreaseHealth()
{
while (currentHealth > 0f)
{
currentHealth -= decreaseSpeed;
UpdateHealthBar();
yield return new WaitForSeconds(1f);
}
// 执行死亡逻辑
}
void UpdateHealthBar()
{
float healthRatio = currentHealth / maxHealth;
healthBar.transform.localScale = new Vector3(healthRatio, 1, 1);
}
}
```
在 Start 方法中启动协程,并在协程中每隔1秒钟减少一定的血量值,然后更新血条的显示。当角色的血量值小于等于0时,协程自动停止。注意,可以根据需要调整减少速度和更新频率。
阅读全文
相关推荐
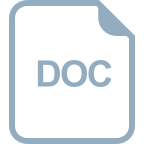
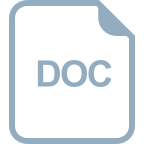


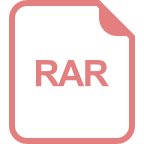
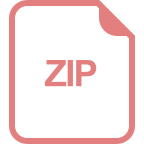
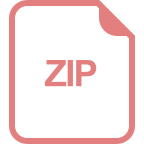
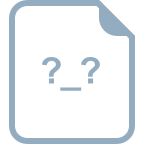
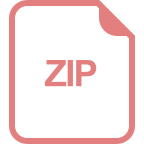








