module.exports = app => { const tutorials = require("../controllers/tutorial.controller.js"); var router = require("express").Router(); // Create a new Tutorial router.post("/", tutorials.create); // Retrieve all Tutorials router.get("/", tutorials.findAll); // Retrieve all published Tutorials router.get("/published", tutorials.findAllPublished); // Retrieve a single Tutorial with id router.get("/:id", tutorials.findOne); // Update a Tutorial with id router.put("/:id", tutorials.update); // Delete a Tutorial with id router.delete("/:id", tutorials.delete); // Delete all Tutorials router.delete("/", tutorials.deleteAll); app.use('/api/tutorials', router); }; 分析一下
时间: 2024-04-19 12:23:34 浏览: 35
这段代码是一个模块导出的函数,接受一个参数app。主要功能是定义了一些路由规则,将这些路由规则绑定到了Express应用程序中。
首先,通过`require`语句引入了一个tutorial控制器,并创建了一个Express的Router实例。
接下来,使用`router.post()`、`router.get()`、`router.put()`、`router.delete()`等方法定义了不同HTTP方法对应的路由规则。这些路由规则会调用tutorial控制器中对应的处理函数。
具体的路由规则如下:
- `router.post("/")`:创建一个新的Tutorial。
- `router.get("/")`:获取所有Tutorials。
- `router.get("/published")`:获取所有已发布的Tutorials。
- `router.get("/:id")`:获取指定id的Tutorial。
- `router.put("/:id")`:更新指定id的Tutorial。
- `router.delete("/:id")`:删除指定id的Tutorial。
- `router.delete("/")`:删除所有Tutorials。
最后,通过`app.use()`方法将上述定义的路由规则绑定到了路径`/api/tutorials`上,即所有以`/api/tutorials`开头的请求都会经过这些路由规则进行处理。
这段代码的作用是将这些路由规则注册到Express应用程序中,以便在收到对应路径的请求时进行相应的处理。
相关问题
module.exports = router //module.exports = app
你的第一个问题是关于代码中的两个不同的 module.exports 赋值语句的区别。其中,第一个赋值语句将 router 对象赋值给 module.exports,而第二个赋值语句将 app 对象赋值给 module.exports。这两个赋值语句的区别在于,router 对象通常用于创建和管理路由,而 app 对象则是整个应用程序的主对象,用于处理 HTTP 请求和响应等任务。因此,如果你的代码中使用了 router 对象来处理路由,那么应该将其赋值给 module.exports。如果你的代码中使用了 app 对象来处理 HTTP 请求和响应等任务,那么应该将其赋值给 module.exports。
module.exports = router module.exports = app可以一起写吗
不能写在一起,因为 `module.exports` 只能赋值一次。当你写了 `module.exports = router` 后,`module.exports` 就只能导出 `router` 对象。如果你写了第二个 `module.exports = app`,它会覆盖前面的赋值,导致只能导出 `app` 对象,而无法导出 `router` 对象。
如果你需要同时导出 `router` 和 `app` 对象,可以将它们放在一个对象中,然后将该对象赋值给 `module.exports`。例如:
```
const express = require('express');
const app = express();
const router = express.Router();
// 定义路由
router.get('/', (req, res) => {
res.send('Hello, world!');
});
// 导出 router 和 app
module.exports = {
router,
app
};
```
这样,你就可以在其他文件中访问 `router` 和 `app` 了。例如:
```
const express = require('express');
const { router, app } = require('./my-module');
// 在 app 中使用 router
app.use('/', router);
// 启动服务器
app.listen(3000, () => {
console.log('Server started on port 3000');
});
```
相关推荐
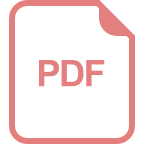
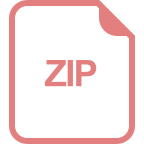
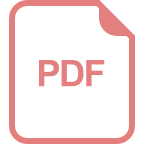












