使用vue3写一个自动获取宽度高度变化的过渡动画
时间: 2024-03-07 14:49:46 浏览: 11
好的,下面是一个使用Vue3编写的自动获取宽度高度变化的过渡动画的示例代码:
```html
<template>
<div class="wrapper" ref="wrapper" v-show="show" :style="{ height: height + 'px' }">
<div class="content" :class="{ animation: animate }">
<slot></slot>
</div>
</div>
</template>
<script>
import { ref, watch, onMounted } from 'vue';
export default {
name: 'AutoAnimate',
props: {
show: {
type: Boolean,
default: false,
},
duration: {
type: Number,
default: 300,
},
},
setup(props) {
const wrapper = ref(null);
const content = ref(null);
const height = ref(0);
const animate = ref(false);
const updateHeight = () => {
height.value = content.value.offsetHeight;
};
watch(
() => props.show,
(newVal, oldVal) => {
if (newVal !== oldVal) {
animate.value = true;
if (newVal) {
content.value.style.opacity = 0;
} else {
content.value.style.opacity = 1;
}
}
}
);
onMounted(() => {
updateHeight();
window.addEventListener('resize', updateHeight);
setTimeout(() => {
animate.value = false;
}, props.duration);
});
return {
wrapper,
content,
height,
animate,
};
},
};
</script>
<style scoped>
.wrapper {
overflow: hidden;
transition: height 300ms;
}
.content {
transition: opacity 300ms;
}
.animation-enter-active,
.animation-leave-active {
transition: opacity 300ms, transform 300ms;
}
.animation-enter,
.animation-leave-to {
opacity: 0;
transform: translateY(-10px);
}
</style>
```
这个组件接受两个属性:`show`和`duration`。`show`用于控制过渡的显示和隐藏,`duration`用于控制过渡动画的持续时间。
在组件的`setup`函数中,我们使用了Vue的`ref`和`watch`函数来监听组件是否显示,并在显示和隐藏时添加过渡动画。同时,我们还使用了`onMounted`函数来在组件挂载时获取初始化高度,并在窗口大小变化时更新高度。
在模板中,我们使用了Vue的`v-show`指令来控制组件的显示和隐藏,并绑定了高度的样式。同时,我们在组件的插槽中放置了需要显示和隐藏的内容,并添加了过渡动画的样式。
最后,我们将这个组件注册为全局组件,就可以在其他组件中使用了:
```js
import AutoAnimate from './AutoAnimate.vue';
app.component('AutoAnimate', AutoAnimate);
```
在其他组件中使用示例:
```html
<template>
<auto-animate :show="show" :duration="500">
<div class="content">
<!-- 需要显示和隐藏的内容 -->
...
</div>
</auto-animate>
</template>
<script>
export default {
name: 'MyComponent',
data() {
return {
show: true,
};
},
};
</script>
```
相关推荐
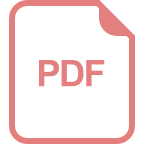
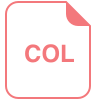
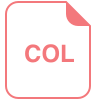
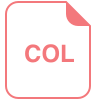
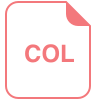
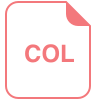







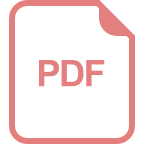
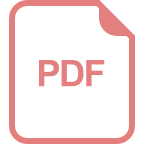