AttributeError: 'Series' object has no attribute 'reshape'
时间: 2023-09-22 14:07:01 浏览: 46
This error occurs when you try to use the reshape method on a pandas Series object. The reshape method is only available for numpy arrays and not for pandas Series.
To fix this error, you should convert the Series object to a numpy array using the values attribute and then use the reshape method on the resulting numpy array.
For example:
```
import pandas as pd
import numpy as np
# Create a pandas Series
s = pd.Series([1, 2, 3, 4, 5])
# Convert the Series to a numpy array and reshape it
arr = s.values.reshape((5, 1))
```
相关问题
出现了报错AttributeError: 'Series' object has no attribute 'reshape'
当出现报错AttributeError: 'Series' object has no attribute 'reshape'时,这意味着你正在尝试在Pandas对象上调用reshape()方法,但是Series对象没有reshape()方法。reshape()方法是用于改变数组的形状的,而是Series对象。
解决这个问题的方法是将Series对象转换为NumPy数组,然后再调用reshape()方法。你可以使用values属性将Series对象转换为NumPy数组,然后再调用reshape()方法。
下面是一个示例代码,演示了如何解决这个问题[^2]:
```python
import pandas as pd
import numpy as np
# 创建一个Series对象
s = pd.Series([1, 2, 3, 4, 5])
# 将Series对象转换为NumPy数组
arr = s.values
# 调用reshape()方法改变数组形状
reshaped_arr = arr.reshape((5, 1))
print(reshaped_arr)
```
这段代码首先创建了一个Series对象s,然使用values属性将其转换为NumPy数组arr。接下来,调用reshape()方法将数组形状改变为(5,1)。最后,打印出改变形状后的数组reshaped_arr。
AttributeError: 'Series' object has no attribute 'reshape'这是什么错误
AttributeError: 'Series' object has no attribute 'reshape'是一个错误提示,意味着在一个Series对象上调用了reshape方法,但是Series对象并没有该方法。Series是pandas库中的一种数据结构,类似于一维数组,而reshape是numpy库中的方法,用于改变数组的形状。
可能的原因是你在使用pandas的Series对象时,错误地调用了numpy的reshape方法。要解决这个问题,你可以考虑以下几点:
1. 确保你导入了正确的库:检查你的代码中是否正确导入了pandas和numpy库。
2. 检查对象类型:确保你调用reshape方法的对象是numpy数组而不是pandas的Series对象。你可以使用type()函数来检查对象的类型。
3. 使用pandas提供的方法:如果你想改变Series对象的形状,可以使用pandas提供的方法,如`Series.values.reshape()`或`Series.to_numpy().reshape()`。
4. 转换为numpy数组:如果你确实需要使用numpy的reshape方法,可以将Series对象转换为numpy数组后再调用该方法,如`Series.to_numpy().reshape()`。
希望以上解答对你有帮助!
相关推荐
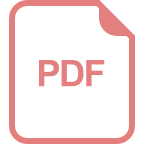
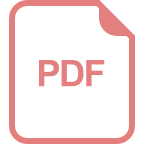
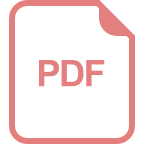












