用c语言将字符串转为数值
时间: 2024-01-01 17:04:18 浏览: 95
可以使用C语言 stdlib.h 库中的 atoi() 或 atof() 函数将字符串转为整数或浮点数。例如:
char str[] = "123";
int num = atoi(str); // 将字符串转为整数,num 的值为 123
char str[] = "3.14";
float num = atof(str); // 将字符串转为浮点数,num 的值为 3.14
相关问题
C语言,将数值转为字符串
C语言是一种强大的系统级编程语言,它具有高效和灵活性的特点,被广泛用于开发操作系统、设备驱动程序等底层软件。对于数值转换成字符串,C语言提供了几个标准库函数来实现:
1. `itoa` 函数(`<stdio.h>`):这个函数在早期的C标准中已不再推荐使用,因为它是非标准的,并且在不同的编译器中可能不可用。不过,如果你的编译器支持,可以用来将整数转换为字符串。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char* itoa(int num, char* str, int base);
```
2. `sprintf` 或 `snprintf` 函数(`<stdio.h>`):这两个函数可以格式化输出到字符串,适用于任意类型的数值转换,包括整数、浮点数等。
```c
char str[64]; // 假设我们有一个足够大的缓冲区
sprintf(str, "%d", num); // 将整数转换为字符串
```
或者
```c
snprintf(str, sizeof(str), "%f", (double)num); // 如果你想转换浮点数
```
3. `strtol` 和 `strtod` 函数(`<stdlib.h>`):这些函数直接从字符串解析数值,但它们不是直接将数值转换成字符串,而是提供了一种从字符串读取数值的方式。
```c
#include <stdlib.h>
#include <string.h>
int main() {
char str[] = "123";
char* endptr;
long int num = strtol(str, &endptr, 10);
if (*endptr == '\0') { // 检查是否成功转换
// 使用num
}
}
```
4. `printf` 函数结合格式化字符 `%s`:如果你只是想打印数值作为字符串,可以直接使用 printf,不需要转换。
```c
printf("The number is: %d\n", num);
```
C++如何将字符串转为数值
C语言中可以使用函数库中的函数将字符串转为数值,例如:
1. atoi()函数:将字符串转为整数。
```c
#include <stdlib.h>
int atoi(const char *str);
```
示例:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char str1[] = "123";
char str2[] = "456.789";
int num1 = atoi(str1);
int num2 = atoi(str2);
printf("%d\n", num1); // 123
printf("%d\n", num2); // 456
return 0;
}
```
2. atof()函数:将字符串转为浮点数。
```c
#include <stdlib.h>
double atof(const char *str);
```
示例:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char str1[] = "123.456";
char str2[] = "789";
double num1 = atof(str1);
double num2 = atof(str2);
printf("%f\n", num1); // 123.456000
printf("%f\n", num2); // 789.000000
return 0;
}
```
3. strtol()函数:将字符串转为长整数。
```c
#include <stdlib.h>
long int strtol(const char *str, char **endptr, int base);
```
示例:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char str1[] = "123";
char str2[] = "0x1A";
char *endptr;
long int num1 = strtol(str1, &endptr, 10);
long int num2 = strtol(str2, &endptr, 16);
printf("%ld\n", num1); // 123
printf("%ld\n", num2); // 26
return 0;
}
```
4. strtod()函数:将字符串转为双精度浮点数。
```c
#include <stdlib.h>
double strtod(const char *str, char **endptr);
```
示例:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char str1[] = "123.456";
char str2[] = "789";
char *endptr;
double num1 = strtod(str1, &endptr);
double num2 = strtod(str2, &endptr);
printf("%f\n", num1); // 123.456000
printf("%f\n", num2); // 789.000000
return 0;
}
```
阅读全文
相关推荐



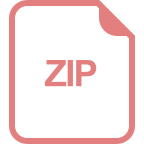
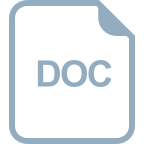






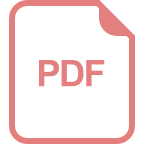
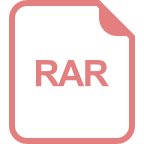
