写一段远程线程注入代码的例子
时间: 2023-04-05 19:05:09 浏览: 95
以下是一段远程线程注入代码的例子:
```
#include <Windows.h>
#include <TlHelp32.h>
DWORD GetProcessIdByName(const wchar_t* processName)
{
DWORD processId = 0;
HANDLE snapshot = CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS, 0);
if (snapshot != INVALID_HANDLE_VALUE)
{
PROCESSENTRY32 processEntry = { 0 };
processEntry.dwSize = sizeof(PROCESSENTRY32);
if (Process32First(snapshot, &processEntry))
{
do
{
if (_wcsicmp(processEntry.szExeFile, processName) == 0)
{
processId = processEntry.th32ProcessID;
break;
}
} while (Process32Next(snapshot, &processEntry));
}
CloseHandle(snapshot);
}
return processId;
}
int main()
{
const wchar_t* targetProcessName = L"notepad.exe";
const wchar_t* dllPath = L"C:\\path\\to\\mydll.dll";
DWORD targetProcessId = GetProcessIdByName(targetProcessName);
if (targetProcessId == 0)
{
printf("Target process not found.\n");
return 1;
}
HANDLE targetProcess = OpenProcess(PROCESS_CREATE_THREAD | PROCESS_QUERY_INFORMATION | PROCESS_VM_OPERATION | PROCESS_VM_WRITE | PROCESS_VM_READ, FALSE, targetProcessId);
if (targetProcess == NULL)
{
printf("Failed to open target process.\n");
return 1;
}
LPVOID dllPathAddress = VirtualAllocEx(targetProcess, NULL, wcslen(dllPath) * sizeof(wchar_t), MEM_COMMIT, PAGE_READWRITE);
if (dllPathAddress == NULL)
{
printf("Failed to allocate memory in target process.\n");
CloseHandle(targetProcess);
return 1;
}
if (!WriteProcessMemory(targetProcess, dllPathAddress, dllPath, wcslen(dllPath) * sizeof(wchar_t), NULL))
{
printf("Failed to write to target process memory.\n");
VirtualFreeEx(targetProcess, dllPathAddress, 0, MEM_RELEASE);
CloseHandle(targetProcess);
return 1;
}
HMODULE kernel32Module = GetModuleHandle(L"kernel32.dll");
if (kernel32Module == NULL)
{
printf("Failed to get kernel32 module handle.\n");
VirtualFreeEx(targetProcess, dllPathAddress, 0, MEM_RELEASE);
CloseHandle(targetProcess);
return 1;
}
LPVOID loadLibraryAddress = GetProcAddress(kernel32Module, "LoadLibraryW");
if (loadLibraryAddress == NULL)
{
printf("Failed to get LoadLibraryW address.\n");
VirtualFreeEx(targetProcess, dllPathAddress, 0, MEM_RELEASE);
CloseHandle(targetProcess);
return 1;
}
HANDLE remoteThread = CreateRemoteThread(targetProcess, NULL, 0, (LPTHREAD_START_ROUTINE)loadLibraryAddress, dllPathAddress, 0, NULL);
if (remoteThread == NULL)
{
printf("Failed to create remote thread.\n");
VirtualFreeEx(targetProcess, dllPathAddress, 0, MEM_RELEASE);
CloseHandle(targetProcess);
return 1;
}
WaitForSingleObject(remoteThread, INFINITE);
VirtualFreeEx(targetProcess, dllPathAddress, 0, MEM_RELEASE);
CloseHandle(remoteThread);
CloseHandle(targetProcess);
printf("Injection succeeded.\n");
return 0;
}
```
请注意,这只是一个示例代码,实际使用时需要根据具体情况进行修改和调整。
阅读全文
相关推荐
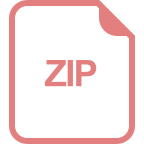
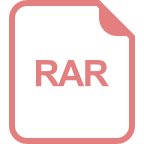
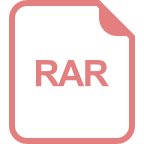















