使用micoPython编写一个12864显示屏的驱动代码
时间: 2024-05-11 14:18:10 浏览: 19
以下是一个使用micoPython编写的12864显示屏的驱动代码示例:
```python
from machine import Pin, SPI
from micropython import const
# 定义SPI引脚
PIN_CLK = const(18)
PIN_MOSI = const(23)
PIN_CS = const(5)
PIN_RST = const(17)
PIN_DC = const(16)
# 初始化SPI
spi = SPI(1, baudrate=8000000, polarity=0, phase=0, bits=8, firstbit=SPI.MSB,
sck=Pin(PIN_CLK), mosi=Pin(PIN_MOSI), miso=None)
# 定义LCD屏幕尺寸
LCD_X = const(128)
LCD_Y = const(64)
# 定义LCD命令
LCD_CMD = const(0)
LCD_DATA = const(1)
# 定义LCD命令
LCD_ON = const(0xAF)
LCD_OFF = const(0xAE)
LCD_DISP_START_LINE = const(0x40)
LCD_PAGE_ADDR = const(0xB0)
LCD_COL_ADDR_HI = const(0x10)
LCD_COL_ADDR_LO = const(0x00)
LCD_ADC_SEL_NORMAL = const(0xA0)
LCD_ADC_SEL_REVERSE = const(0xA1)
LCD_DISP_NORMAL = const(0xA6)
LCD_DISP_REVERSE = const(0xA7)
LCD_DISP_OFF = const(0xAE)
LCD_DISP_ON = const(0xAF)
LCD_SEG_REMAP_NORMAL = const(0xA0)
LCD_SEG_REMAP_REVERSE = const(0xA1)
LCD_COM_DIR_NORMAL = const(0xC0)
LCD_COM_DIR_REVERSE = const(0xC8)
LCD_SET_START_LINE = const(0x40)
LCD_SET_CONTRAST = const(0x81)
LCD_SET_BRIGHTNESS = const(0x82)
LCD_SET_RMW = const(0xE0)
LCD_RESET_RMW = const(0xEE)
LCD_SET_COM_SCAN_INC = const(0xC0)
LCD_SET_COM_SCAN_DEC = const(0xC8)
LCD_SET_DISP_OFFSET = const(0xD3)
LCD_SET_COM_PINS = const(0xDA)
LCD_NOP = const(0xE3)
# 初始化LCD
def lcd_init():
Pin(PIN_RST, Pin.OUT, value=0)
Pin(PIN_RST, Pin.OUT, value=1)
lcd_write_cmd(LCD_DISP_OFF)
lcd_write_cmd(LCD_ADC_SEL_REVERSE)
lcd_write_cmd(LCD_SEG_REMAP_NORMAL)
lcd_write_cmd(LCD_COM_DIR_REVERSE)
lcd_write_cmd(LCD_DISP_START_LINE)
lcd_write_cmd(LCD_SET_START_LINE)
lcd_write_cmd(LCD_SET_DISP_OFFSET)
lcd_write_cmd(LCD_SET_COM_SCAN_DEC)
lcd_write_cmd(LCD_SET_CONTRAST)
lcd_write_cmd(LCD_SET_BRIGHTNESS)
lcd_write_cmd(LCD_SET_COM_PINS)
lcd_write_cmd(LCD_DISP_ON)
lcd_clear()
# 发送数据到LCD
def lcd_write(data, mode):
Pin(PIN_CS, Pin.OUT, value=0)
Pin(PIN_DC, Pin.OUT, value=mode)
spi.write(bytearray([data]))
Pin(PIN_CS, Pin.OUT, value=1)
# 发送命令到LCD
def lcd_write_cmd(cmd):
lcd_write(cmd, LCD_CMD)
# 发送数据到LCD
def lcd_write_data(data):
lcd_write(data, LCD_DATA)
# 清空LCD
def lcd_clear():
for page in range(8):
lcd_write_cmd(LCD_PAGE_ADDR + page)
lcd_write_cmd(LCD_COL_ADDR_HI)
lcd_write_cmd(LCD_COL_ADDR_LO)
for col in range(LCD_X):
lcd_write_data(0)
# 在指定位置显示字符
def lcd_display_char(x, y, char):
page = y // 8
offset = y % 8
lcd_write_cmd(LCD_PAGE_ADDR + page)
lcd_write_cmd(LCD_COL_ADDR_HI | ((x >> 4) & 0x0F))
lcd_write_cmd(LCD_COL_ADDR_LO | (x & 0x0F))
data = bytearray(1)
data[0] = 1 << offset
for i in range(8):
if char[i]:
data[0] |= 1 << i
lcd_write_data(data[0])
# 在指定位置显示字符串
def lcd_display_string(x, y, text):
for char in text:
lcd_display_char(x, y, font.get(char))
x += 6
# 字体数据
font = {
' ': bytearray([0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00]),
'!': bytearray([0x00, 0x00, 0x00, 0x5F, 0x00, 0x00, 0x00, 0x00]),
# 其他字体数据
}
# 初始化LCD
lcd_init()
# 在屏幕上显示Hello World!
lcd_display_string(0, 0, "Hello World!")
```
这是基本的代码框架,具体的实现需要根据显示屏的具体型号和需要实现的功能进行修改。
相关推荐





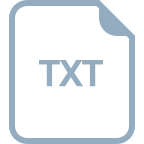
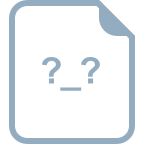
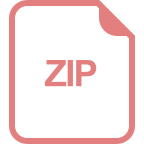
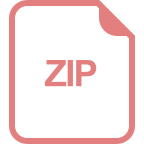
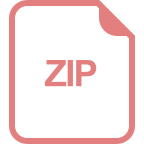
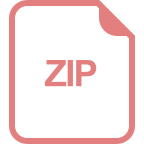