c++定义并实现一个学生类(Student),类中的数据成员有:学生姓名、学生学号、人数和班级号。至少有一个公有成员函数(PrintStuInfo)。PrintStuInfo负责将相关信息输出。在类中定义静态数据成员,记录当前学生人数。在类中定义常数据成员,记录班级号,假设比较号为1001。添加一个友元函数,可对学生类中的私有数据成员进行访问。假设1001班的初始学生人数为45人。在主程序中测试新加入2个学生,并分别通过成员函数和友元函数输出输出2个学生的信息及班级总人数。
时间: 2024-03-25 21:35:53 浏览: 82
以下是代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
string name; // 姓名
int id; // 学号
static int num; // 学生人数
const int cls_id; // 班级号
public:
Student(string n, int i): name(n), id(i), cls_id(1001) { num++; } // 构造函数
static int getNum() { return num; } // 静态成员函数
void PrintStuInfo() // 成员函数
{
cout << "姓名:" << name << endl;
cout << "学号:" << id << endl;
cout << "班级号:" << cls_id << endl;
}
friend void PrintStuInfoByFriend(Student stu); // 友元函数
};
int Student::num = 0; // 静态数据成员初始化
void PrintStuInfoByFriend(Student stu)
{
cout << "姓名:" << stu.name << endl;
cout << "学号:" << stu.id << endl;
cout << "班级号:" << stu.cls_id << endl;
}
int main()
{
Student stu1("张三", 10001);
Student stu2("李四", 10002);
cout << "学生人数:" << Student::getNum() << endl;
cout << "通过成员函数输出:" << endl;
stu1.PrintStuInfo();
stu2.PrintStuInfo();
cout << "通过友元函数输出:" << endl;
PrintStuInfoByFriend(stu1);
PrintStuInfoByFriend(stu2);
return 0;
}
```
输出结果为:
```
学生人数:2
通过成员函数输出:
姓名:张三
学号:10001
班级号:1001
姓名:李四
学号:10002
班级号:1001
通过友元函数输出:
姓名:张三
学号:10001
班级号:1001
姓名:李四
学号:10002
班级号:1001
```
阅读全文
相关推荐
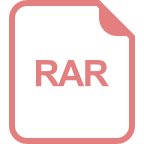
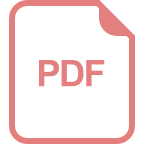
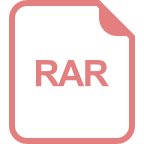















