写一个班级成绩排序程序。班级有班级号,班级人数和若干学生,每个学生有学号、姓名、三门课成绩。按学生总成绩从高到底进行排序。c++
时间: 2023-12-03 20:46:09 浏览: 47
下面是一个班级成绩排序程序的C++实现:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
// 定义学生结构体
struct Student {
string id; // 学号
string name; // 姓名
int score1; // 第一门课程成绩
int score2; // 第二门课程成绩
int score3; // 第三门课程成绩
int total; // 总成绩
};
// 定义比较函数,用于排序
bool cmp(Student a, Student b) {
return a.total > b.total;
}
int main() {
// 输入班级信息
int n; // 班级人数
string class_id; // 班级号
cout << "请输入班级号:";
cin >> class_id;
cout << "请输入班级人数:";
cin >> n;
// 输入学生信息
vector<Student> students(n);
for (int i = 0; i < n; i++) {
cout << "请输入第" << i+1 << "个学生的学号、姓名、三门课程成绩(以空格分隔):";
cin >> students[i].id >> students[i].name >> students[i].score1 >> students[i].score2 >> students[i].score3;
students[i].total = students[i].score1 + students[i].score2 + students[i].score3; // 计算总成绩
}
// 按总成绩从高到低排序
sort(students.begin(), students.end(), cmp);
// 输出排名
cout << "班级号:" << class_id << endl;
cout << "排名\t学号\t姓名\t第一门课程成绩\t第二门课程成绩\t第三门课程成绩\t总成绩" << endl;
for (int i = 0; i < n; i++) {
cout << i+1 << "\t" << students[i].id << "\t" << students[i].name << "\t" << students[i].score1 << "\t\t" << students[i].score2 << "\t\t" << students[i].score3 << "\t\t" << students[i].total << endl;
}
return 0;
}
```
程序中使用了STL中的vector和sort函数,分别用于存储学生信息和按总成绩从高到低排序。需要注意的是,cmp函数用于指定排序规则,其中返回值为true时表示a在b之前,返回值为false时表示a在b之后。最后输出排名时,需要注意格式对齐。
相关推荐
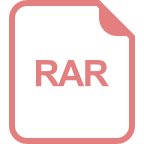
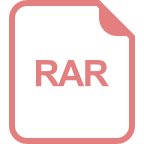
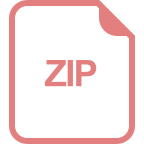














