用C++写一个学生管理系统要求有学号,姓名,成绩,班级
时间: 2023-05-12 09:03:15 浏览: 236
好的,这个问题很容易,以下是一个简单的学生管理系统的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENTS 100
struct student {
int id;
char name[50];
int score;
char class[20];
};
struct student students[MAX_STUDENTS];
int num_students = 0;
void add_student() {
if (num_students >= MAX_STUDENTS) {
printf("Error: too many students\n");
return;
}
struct student s;
printf("Enter student ID: ");
scanf("%d", &s.id);
printf("Enter student name: ");
scanf("%s", s.name);
printf("Enter student score: ");
scanf("%d", &s.score);
printf("Enter student class: ");
scanf("%s", s.class);
students[num_students++] = s;
printf("Student added successfully\n");
}
void list_students() {
printf("ID\tName\tScore\tClass\n");
for (int i = 0; i < num_students; i++) {
printf("%d\t%s\t%d\t%s\n", students[i].id, students[i].name, students[i].score, students[i].class);
}
}
int main() {
while (1) {
printf("Enter command (add, list, quit): ");
char command[10];
scanf("%s", command);
if (strcmp(command, "add") == 0) {
add_student();
} else if (strcmp(command, "list") == 0) {
list_students();
} else if (strcmp(command, "quit") == 0) {
break;
} else {
printf("Unknown command: %s\n", command);
}
}
return 0;
}
```
这个程序使用了一个结构体来存储每个学生的信息,包括学号、姓名、成绩和班级。它还使用了一个数组来存储所有学生的信息。程序提供了三个命令:add(添加学生)、list(列出所有学生)和quit(退出程序)。
阅读全文
相关推荐
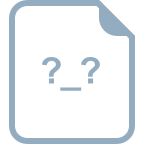
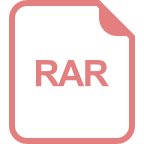
















