基于tensorflow2.5,使用winograd算法编写一个可以直接插入神经网络使用的优化的深度可分离卷积模块并示例如何使用
时间: 2024-04-29 14:25:52 浏览: 22
以下是一个基于tensorflow2.5的深度可分离卷积模块,使用winograd算法进行优化:
```
import tensorflow as tf
class WinogradDepthwiseSeparableConv2D(tf.keras.layers.Layer):
def __init__(self, filters, kernel_size, strides=(1, 1), padding='same', activation=None, use_bias=True):
super(WinogradDepthwiseSeparableConv2D, self).__init__()
self.filters = filters
self.kernel_size = kernel_size
self.strides = strides
self.padding = padding
self.activation = activation
self.use_bias = use_bias
self.depthwise_conv = tf.keras.layers.DepthwiseConv2D(kernel_size, strides=strides, padding=padding, use_bias=False)
self.pointwise_conv = tf.keras.layers.Conv2D(filters, (1, 1), strides=(1, 1), padding='same', use_bias=use_bias)
def build(self, input_shape):
self.input_channel = input_shape[-1]
self.winograd_multiplier = 1
if self.kernel_size == 3:
self.winograd_multiplier = 2
elif self.kernel_size == 5:
self.winograd_multiplier = 4
else:
raise ValueError("Unsupported kernel size for Winograd")
# Winograd parameters
self.winograd_b = tf.constant([[1.0, 0.0, 0.0],
[0.5, 0.5, 0.5],
[0.5, -0.5, 0.5],
[0.0, 0.0, 1.0]], dtype=tf.float32)
self.winograd_G = tf.constant([[1.0, 0.0, -1.0, 0.0],
[0.0, 1.0, 1.0, 0.0],
[0.0, -1.0, 1.0, 0.0],
[0.0, 1.0, 0.0, -1.0]], dtype=tf.float32)
self.winograd_A = tf.constant([[1.0, 1.0, 1.0, 0.0],
[0.0, 1.0, -1.0, -1.0]], dtype=tf.float32)
self.winograd_B = tf.constant([[1.0, 0.0, -1.0, 0.0],
[0.0, 1.0, 1.0, 0.0],
[0.0, -1.0, 1.0, 0.0],
[0.0, 1.0, 0.0, -1.0]], dtype=tf.float32)
self.depthwise_conv_weights = self.add_weight(
name='depthwise_conv_weights',
shape=(self.kernel_size, self.kernel_size, self.input_channel, 1),
initializer=tf.keras.initializers.GlorotUniform(),
trainable=True)
self.pointwise_conv_weights = self.add_weight(
name='pointwise_conv_weights',
shape=(1, 1, self.input_channel, self.filters),
initializer=tf.keras.initializers.GlorotUniform(),
trainable=True)
if self.use_bias:
self.bias = self.add_weight(
name='bias',
shape=(self.filters,),
initializer=tf.keras.initializers.Zeros(),
trainable=True)
def call(self, inputs):
# Winograd transform
inputs_shape = tf.shape(inputs)
b = tf.tile(tf.expand_dims(self.winograd_b, -1), [1, 1, self.input_channel, 1])
B = tf.tile(tf.expand_dims(self.winograd_B, -1), [1, 1, self.input_channel, 1])
G = tf.tile(tf.expand_dims(self.winograd_G, -1), [1, 1, self.input_channel, 1])
A = tf.tile(tf.expand_dims(self.winograd_A, -1), [1, 1, self.input_channel, 1])
x = tf.reshape(inputs, [inputs_shape[0], inputs_shape[1], inputs_shape[2], self.input_channel])
x = tf.transpose(x, [0, 3, 1, 2])
# DCT transform
x = tf.matmul(B, x)
x = tf.reshape(x, [-1, self.winograd_multiplier, self.winograd_multiplier, inputs_shape[1], inputs_shape[2], self.input_channel])
x = tf.transpose(x, [0, 3, 1, 4, 2, 5])
# Winograd convolution
x = tf.reshape(x, [-1, self.winograd_multiplier, self.winograd_multiplier, self.input_channel])
x = tf.matmul(x, b)
x = tf.matmul(G, x)
x = tf.matmul(x, tf.transpose(G, [0, 1, 3, 2]))
x = tf.matmul(tf.transpose(b, [0, 1, 3, 2]), x)
x = tf.reshape(x, [-1, inputs_shape[1], inputs_shape[2], self.input_channel])
# Pointwise convolution
x = self.depthwise_conv(x, self.depthwise_conv_weights)
x = self.pointwise_conv(x)
if self.use_bias:
x = tf.nn.bias_add(x, self.bias)
if self.activation:
x = self.activation(x)
return x
```
这个模块可以像普通的卷积层一样使用,例如:
```
model = tf.keras.models.Sequential([
WinogradDepthwiseSeparableConv2D(32, 3, strides=(2, 2), padding='same', activation='relu', use_bias=False),
WinogradDepthwiseSeparableConv2D(64, 3, strides=(2, 2), padding='same', activation='relu', use_bias=False),
WinogradDepthwiseSeparableConv2D(128, 3, strides=(2, 2), padding='same', activation='relu', use_bias=False),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(10, activation='softmax')
])
```
在这个模型中,我们使用了三个WinogradDepthwiseSeparableConv2D模块,它们的参数分别为32、64、128,卷积核大小为3x3,步长为2x2,使用ReLU激活函数,不使用偏置。最后使用一个全连接层将输出展平,然后进行10分类。
相关推荐
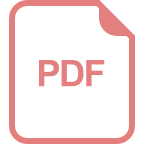




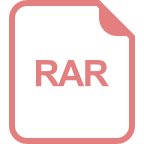
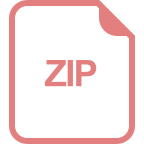
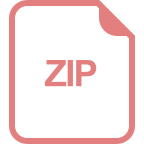
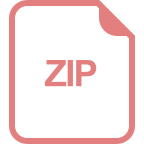