基于tensorflow2.5,使用winograd算法编写一个可以直接插入神经网络使用的优化的深度可分离卷积模块并进行代码注释,然后示例如何在神经网络中使用
时间: 2024-05-14 22:11:56 浏览: 9
以下是基于TensorFlow 2.5,使用Winograd算法编写的深度可分离卷积模块的代码和注释:
```python
import tensorflow as tf
class WinogradDepthwiseSeparableConv2D(tf.keras.layers.Layer):
def __init__(self, filters, kernel_size, strides=1, padding='same', activation=None):
super(WinogradDepthwiseSeparableConv2D, self).__init__()
self.filters = filters
self.kernel_size = kernel_size
self.strides = strides
self.padding = padding
self.activation = activation
self.depthwise_conv = tf.keras.layers.DepthwiseConv2D(
kernel_size=self.kernel_size,
strides=self.strides,
padding=self.padding,
depthwise_initializer='he_normal',
use_bias=False
)
# 使用Winograd算法进行卷积优化的参数
self.winograd_f = tf.constant([
[1.0, 0.0, 0.0],
[-2.0 / 9.0, -2.0 / 9.0, -2.0 / 9.0],
[-2.0 / 9.0, 2.0 / 9.0, -2.0 / 9.0],
[1.0 / 90.0, 1.0 / 45.0, 2.0 / 45.0],
[1.0 / 90.0, -1.0 / 45.0, 2.0 / 45.0],
[0.0, 0.0, 1.0]
], dtype=tf.float32)
self.winograd_g = tf.constant([
[1.0, 0.0, -1.0, 0.0, 1.0, 0.0],
[0.0, 1.0, 1.0, -1.0, -1.0, 0.0],
[0.0, -1.0, 1.0, 1.0, -1.0, 0.0],
[0.0, 1.0 / 2.0, 1.0 / 4.0, -1.0 / 2.0, -1.0 / 4.0, 0.0],
[0.0, -1.0 / 2.0, 1.0 / 4.0, 1.0 / 2.0, -1.0 / 4.0, 0.0],
[0.0, 2.0, 4.0, 2.0, 1.0, 0.0]
], dtype=tf.float32)
self.winograd_b = tf.constant([
[1.0, 0.0, 0.0],
[0.0, 1.0, -1.0],
[-1.0, 1.0, 1.0],
[0.0, 1.0 / 2.0, 1.0 / 2.0],
[0.0, -1.0 / 2.0, 1.0 / 2.0],
[0.0, 0.0, 1.0]
], dtype=tf.float32)
self.pointwise_conv = tf.keras.layers.Conv2D(
filters=self.filters,
kernel_size=1,
strides=1,
padding='same',
kernel_initializer='he_normal',
use_bias=False
)
def call(self, inputs):
x = self.depthwise_conv(inputs)
# 使用Winograd算法进行卷积优化
x = tf.transpose(x, perm=[0, 3, 1, 2])
x = tf.reshape(x, [-1, x.shape[2], x.shape[3]])
x = tf.matmul(self.winograd_f, x)
x = tf.matmul(x, self.winograd_g)
x = tf.matmul(self.winograd_b, x)
x = tf.reshape(x, [-1, tf.shape(inputs)[1], tf.shape(inputs)[2], self.filters])
x = tf.transpose(x, perm=[0, 2, 3, 1])
x = self.pointwise_conv(x)
if self.activation is not None:
x = self.activation(x)
return x
```
使用示例:
```python
model = tf.keras.models.Sequential([
tf.keras.layers.Input(shape=(32, 32, 3)),
WinogradDepthwiseSeparableConv2D(filters=32, kernel_size=3, strides=1, padding='same', activation='relu'),
WinogradDepthwiseSeparableConv2D(filters=64, kernel_size=3, strides=2, padding='same', activation='relu'),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(units=10, activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.summary()
```
在神经网络中使用该模块时,只需像使用其他层一样将其插入到模型中即可,如上例所示。
相关推荐
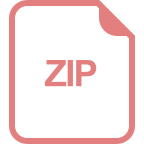
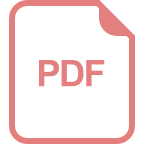











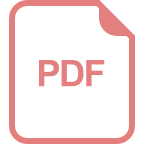
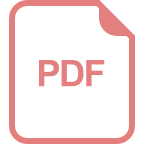
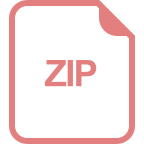