用Java写一个坦克大战游戏
时间: 2023-12-19 09:04:46 浏览: 24
坦克大战是一款经典的游戏,下面是一个使用Java编写的简单的坦克大战游戏的示例。
首先,你需要创建一个Tank类来表示坦克,这个类应该包含坦克的位置、方向、速度等信息,以及坦克的移动、射击等方法。
```java
public class Tank {
private int x; // 坦克的横坐标
private int y; // 坦克的纵坐标
private int direction; // 坦克的方向(0:上,1:下,2:左,3:右)
private int speed; // 坦克的速度
public Tank(int x, int y, int direction, int speed) {
this.x = x;
this.y = y;
this.direction = direction;
this.speed = speed;
}
public void moveUp() {
y -= speed;
}
public void moveDown() {
y += speed;
}
public void moveLeft() {
x -= speed;
}
public void moveRight() {
x += speed;
}
public void fire() {
// 射击的逻辑代码
}
// 其他方法
}
```
然后,你需要创建一个Game类来控制游戏的逻辑,包括创建坦克、敌人、地图等元素,并实现游戏的循环逻辑。
```java
public class Game {
private Tank playerTank; // 玩家坦克
private List<Tank> enemyTanks; // 敌人坦克列表
private int[][] map; // 地图
private boolean gameOver; // 游戏是否结束
public Game() {
// 初始化地图、玩家坦克、敌人坦克等
}
public void run() {
while (!gameOver) {
// 处理用户输入
// 移动玩家坦克
// 移动敌人坦克
// 判断是否有坦克死亡等逻辑
// 绘制游戏界面
}
}
// 其他方法
}
```
最后,你可以在游戏主方法中创建一个Game对象并运行游戏。
```java
public static void main(String[] args) {
Game game = new Game();
game.run();
}
```
以上是一个简单的坦克大战游戏的示例,你可以根据自己的需求和想象进行适当的修改和扩展。
相关推荐
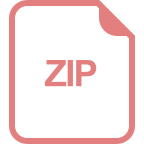
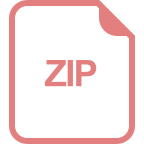








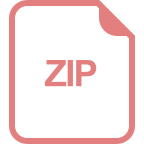
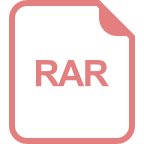
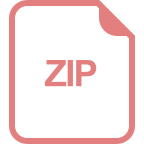
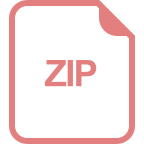
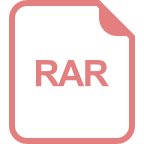