dcmSOPs = findSOPs(path) paths = list(map(str,path.split(""))) patient = paths[-2] time = paths[-1] rvFile = path+'\RS.{}'.format(patient)+'.CT_1.dcm'.format(time) ds = pydicom.dcmread(rvFile) contours = ds.ROIContourSequence dcmFile = path+'\CT.{}'.format(patient)+'.Image {}.dcm'.format(str(int(1))) ds = pydicom.dcmread(dcmFile) dcmOrigin = ds.ImagePositionPatient dcmSpacing = ds.PixelSpacing numberOfContours = len(contours[labelID].ContourSequence) print("time",time,": numberOfContourPts=",numberOfContours) cuts = [] 用中文按行解释
时间: 2024-04-26 09:21:38 浏览: 91
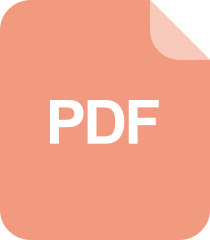
Pro Java 7 NIO.2.pdf
这段代码主要是对 DICOM 文件进行操作的。首先,通过调用函数 `findSOPs` 找到路径为 `path` 的 DICOM 文件的 SOP 序列。然后,将路径 `path` 按照 `'/'` 分割成一个列表 `paths`。其中,`paths[-2]` 表示病人信息,`paths[-1]` 表示时间信息。接着,根据病人信息和时间信息组成 ROI 文件的路径 `rvFile`,并且读取该文件中的 ROIContourSequence。之后,根据病人信息组成 CT 文件的路径 `dcmFile`,并且读取该文件中的 ImagePositionPatient 和 PixelSpacing。最后,根据给定的标签 ID `labelID`,获取对应轮廓的 ContourSequence 中的点的数量,并且将结果存入变量 `numberOfContours`。最后,将空列表 `cuts` 返回。
阅读全文
相关推荐
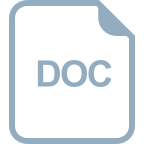
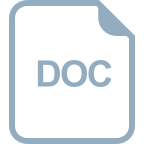


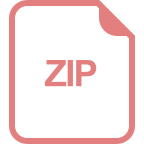
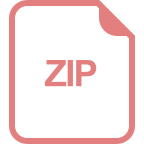
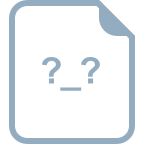
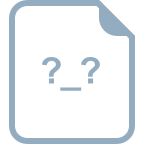
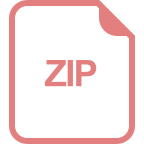
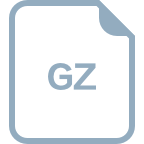
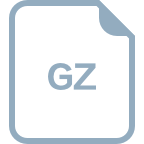
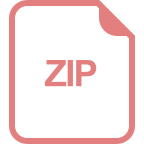
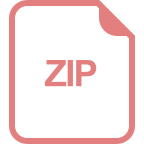
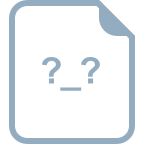
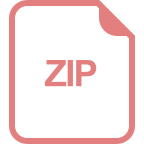
