QML学习笔记(七)— 实现可拖拽、编辑、选中的ListView
时间: 2023-11-26 17:03:22 浏览: 470
实现可拖拽、编辑、选中的ListView可以提升用户交互体验,下面介绍一种实现方法。
1. 定义ListView
首先,我们需要定义一个ListView,用于显示列表项。在ListView中,我们需要指定model、delegate、highlight、onPressed、onPositionChanged等属性。
```
ListView {
id: listView
model: ListModel {
id: listModel
}
delegate: Component {
id: delegate
Rectangle {
id: item
width: listView.width
height: 50
color: "white"
border.width: 1
border.color: "black"
Text {
id: text
anchors.centerIn: parent
text: model.text
}
MouseArea {
id: mouseArea
anchors.fill: parent
drag.target: item
drag.axis: Drag.XAxis
drag.minimumX: -listView.width
drag.maximumX: listView.width
drag.active: listView.currentIndex === index
onPressed: {
listView.currentIndex = index
listView.positionViewAtIndex(index, ListView.Center)
}
}
Rectangle {
id: indicator
width: 10
height: 10
color: "green"
radius: width / 2
visible: listView.currentIndex === index
anchors {
right: parent.right
verticalCenter: parent.verticalCenter
margins: 10
}
}
}
}
highlight: Rectangle {
color: "lightgray"
width: listView.width
height: 50
border.width: 1
border.color: "black"
}
onPositionChanged: {
if (listView.moving) return;
var index = listView.indexAt(listView.contentItem.x, listView.contentItem.y)
if (index !== -1 && index !== listView.currentIndex) {
listView.currentIndex = index
}
}
}
```
在上面的代码中,ListView中的delegate定义了每个列表项的展示方式。我们在delegate中定义了一个MouseArea,用于实现可拖拽的功能。在MouseArea的drag.target属性中,我们指定了可拖拽的目标为列表项item。设置drag.axis为Drag.XAxis,表示只能在水平方向上拖拽。设置drag.minimumX为-listView.width,drag.maximumX为listView.width,表示只能在ListView的宽度范围内拖拽。在onPressed事件中,我们设置了当前列表项为选中状态,并将其居中展示。
2. 定义编辑界面
为了实现编辑功能,我们需要定义一个编辑界面。在编辑界面中,用户可以修改列表项的文本内容。我们可以使用一个Dialog来实现编辑界面。
```
Dialog {
id: editDialog
title: "Edit Item"
modal: true
width: 400
height: 200
visible: false
TextField {
id: textField
anchors.centerIn: parent
width: parent.width - 40
placeholderText: "Enter item text"
}
Button {
text: "OK"
onClicked: {
var index = listView.currentIndex
if (index !== -1) {
listModel.setProperty(index, "text", textField.text)
}
editDialog.close()
}
enabled: textField.text !== ""
anchors {
right: parent.right
bottom: parent.bottom
margins: 10
}
}
Button {
text: "Cancel"
onClicked: editDialog.close()
anchors {
left: parent.left
bottom: parent.bottom
margins: 10
}
}
}
```
在上面的代码中,我们定义了一个Dialog,其中包含一个TextField和两个Button。用户可以在TextField中输入新的文本内容,然后点击OK按钮保存修改,或点击Cancel按钮取消修改。
3. 实现选中效果
为了让用户能够明显地看到当前选中的列表项,我们可以在每个列表项上添加一个指示器。在ListView的delegate中,我们定义了一个Rectangle,用于显示当前选中的列表项。在Rectangle的visible属性中,我们使用listView.currentIndex === index来判断当前列表项是否被选中。
4. 实现编辑功能
在ListView中,我们可以通过双击或长按来触发编辑功能。在ListView的delegate中,我们为item和mouseArea分别定义了onDoubleClicked和onLongPressed事件。在onDoubleClicked事件中,我们弹出编辑界面,让用户可以修改当前选中的列表项的文本内容。在onLongPressed事件中,我们弹出上下文菜单,让用户可以选择删除当前选中的列表项。
```
MouseArea {
id: mouseArea
anchors.fill: parent
drag.target: item
drag.axis: Drag.XAxis
drag.minimumX: -listView.width
drag.maximumX: listView.width
drag.active: listView.currentIndex === index
onPressed: {
listView.currentIndex = index
listView.positionViewAtIndex(index, ListView.Center)
}
onDoubleClicked: {
editDialog.visible = true
textField.text = model.text
textField.selectAll()
}
onLongPressed: {
var menu = Menu {
MenuItem {
text: "Delete"
onTriggered: listModel.remove(index)
}
}
menu.popup()
}
}
```
在上面的代码中,我们为mouseArea分别定义了onDoubleClicked和onLongPressed事件。在onDoubleClicked事件中,我们弹出编辑界面editDialog,并将当前选中的列表项的文本内容显示在TextField中。在onLongPressed事件中,我们弹出上下文菜单Menu,其中包含一个MenuItem,用于删除当前选中的列表项。
5. 实现拖拽功能
在ListView中,我们可以通过拖拽来调整列表项的顺序。在ListView的delegate中,我们为item定义了onDropped事件。在onDropped事件中,我们交换拖拽的两个列表项的位置。
```
Rectangle {
id: item
width: listView.width
height: 50
color: "white"
border.width: 1
border.color: "black"
Text {
id: text
anchors.centerIn: parent
text: model.text
}
MouseArea {
id: mouseArea
anchors.fill: parent
drag.target: item
drag.axis: Drag.XAxis
drag.minimumX: -listView.width
drag.maximumX: listView.width
drag.active: listView.currentIndex === index
onPressed: {
listView.currentIndex = index
listView.positionViewAtIndex(index, ListView.Center)
}
onDropped: {
if (drag.source !== drag.target) {
var fromIndex = drag.source.index
var toIndex = drag.target.index
listModel.move(fromIndex, toIndex, 1)
}
}
}
Rectangle {
id: indicator
width: 10
height: 10
color: "green"
radius: width / 2
visible: listView.currentIndex === index
anchors {
right: parent.right
verticalCenter: parent.verticalCenter
margins: 10
}
}
}
```
在上面的代码中,我们为mouseArea定义了onDropped事件。在onDropped事件中,我们使用ListModel的move方法来交换拖拽的两个列表项的位置。
至此,我们已经实现了可拖拽、编辑、选中的ListView。这个ListView可以让用户方便地调整列表项的顺序,同时也可以让用户快速修改列表项的文本内容。
阅读全文
相关推荐


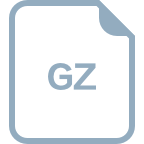












