QML 通过LIstVIew 实现表格,包括表头,表头可调整,列可固定,行可选中。
时间: 2023-10-23 12:12:42 浏览: 281
要通过 QML 的 ListView 实现表格,包括表头、表头可调整、列可固定、行可选中,可以按照以下步骤进行操作:
1. 定义一个 ListView 控件作为表格,设置其大小和位置。
2. 在 ListView 中添加一个 headerItem 控件,用于实现表头。通过设置该控件的大小、位置和内容,可以实现表头的显示。
3. 在 ListView 的 delegate 中定义一个 rowItem 控件,用于实现表格中的每一行。通过设置该控件的大小、位置和内容,可以实现表格中每一行的显示。
4. 在 rowItem 控件中添加一些属性,例如:background、highlight、selected 等,以控制行的背景、高亮和选中状态。
5. 为 headerItem 和 rowItem 添加一些列控件,用于实现每一列的显示。通过设置这些列控件的大小、位置和内容,可以实现每一列的显示。
6. 在列控件中添加一些属性,例如:resizable、fixed 等,以控制列的调整和固定状态。
以下是一个简单的示例代码,用于实现一个包含表头、可调整表头、可固定列和可选中行的表格:
```
import QtQuick 2.0
ListView {
width: 400
height: 300
// 表头
headerItem: Item {
width: parent.width
height: 30
Rectangle {
anchors.fill: parent
color: "lightgray"
Text {
anchors.centerIn: parent
text: "Header 1"
}
}
}
// 每一行
delegate: Rectangle {
width: parent.width
height: 30
// 背景和高亮
property bool highlight: false
property bool selected: false
color: highlight ? "lightblue" : "white"
Rectangle {
anchors.fill: parent
color: selected ? "blue" : "transparent"
opacity: selected ? 0.2 : 0
}
// 每一列
Rectangle {
width: 100
height: parent.height
color: "white"
Text {
anchors.centerIn: parent
text: "Column 1"
}
// 调整和固定
property bool resizable: true
property bool fixed: false
MouseArea {
anchors.fill: parent
cursorShape: resizable ? Qt.SizeHorCursor : fixed ? Qt.ForbiddenCursor : Qt.ArrowCursor
onPressed: {
if (resizable) {
// 调整列宽
console.log("Resize column")
} else if (!fixed) {
// 固定列
console.log("Fix column")
}
}
}
}
// 选中行
MouseArea {
anchors.fill: parent
onClicked: {
selected = !selected
}
}
}
// 数据模型
model: ListModel {
ListElement { name: "Row 1" }
ListElement { name: "Row 2" }
ListElement { name: "Row 3" }
ListElement { name: "Row 4" }
}
}
```
该代码定义了一个大小为 400x300 的 ListView 控件,其中包含了一个 headerItem 控件和一个 delegate 控件。headerItem 控件用于实现表头,通过一个颜色为灰色的矩形和一个显示文本的 Text 控件实现。delegate 控件用于实现表格中的每一行,其中包含了一个大小为 100x30 的 Rectangle 控件,用于实现每一列的显示。通过一个 MouseArea 控件实现列的调整和固定功能,以及一个 MouseArea 控件实现行的选中功能。同时,该代码还定义了一个 ListModel 数据模型,用于存储表格中的数据。
相关推荐
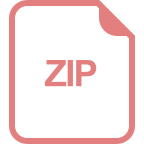
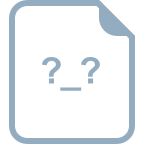














